Optimizing Performance in Ruby: Techniques and Best Practices
When developing Ruby applications, optimizing performance is crucial for delivering fast and efficient software. Whether you’re working on a web application, a script, or a complex algorithm, optimizing your Ruby code can significantly improve execution speed and resource usage. In this blog, we’ll explore various techniques and best practices for optimizing Ruby performance. From efficient data structures to avoiding common pitfalls, we’ll cover a range of strategies to help you achieve optimal performance in your Ruby applications.
Profiling and Benchmarking
Profiling and benchmarking your code are essential steps in identifying performance bottlenecks and measuring improvements. Ruby provides several powerful tools for this purpose, such as the built-in profiler and external libraries like ‘benchmark-ips.’ Let’s see an example of how to use the profiler:
ruby require 'profile' def expensive_operation # Code to be profiled end # Profile the expensive operation profile_result = RubyProf.profile do expensive_operation end # Print the profiling result printer = RubyProf::FlatPrinter.new(profile_result) printer.print(STDOUT)
Efficient Data Structures
Choosing the right data structure can significantly impact performance. Ruby offers various built-in data structures, each with its own advantages and trade-offs. For example, using an Array for large collections where constant-time access is required is more efficient than using a LinkedList. Let’s consider an example using a Hash for efficient lookup:
ruby # Inefficient lookup using an array arr = ['John', 'Jane', 'Michael', 'Sarah'] arr.include?('Michael') #=> true # Efficient lookup using a hash hash = {'John' => true, 'Jane' => true, 'Michael' => true, 'Sarah' => true} hash.key?('Michael') #=> true
Memory Management
Ruby’s garbage collector handles memory management automatically, but there are still techniques to optimize memory usage. Minimizing object allocations, reducing the number of temporary objects, and using symbols instead of strings for keys can help improve memory efficiency. Here’s an example of symbol usage:
ruby # Inefficient string keys user_details = { 'name' => 'John', 'age' => 30, 'email' => 'john@example.com' } # Efficient symbol keys user_details = { name: 'John', age: 30, email: 'john@example.com' }
Optimizing Loops and Iteration
Loops and iteration are fundamental in Ruby programming, and optimizing them can have a significant impact on performance. Using efficient iteration methods like each, map, and reduce instead of traditional loops can improve code readability and performance. Here’s an example:
ruby # Traditional loop numbers = [1, 2, 3, 4, 5] sum = 0 for number in numbers sum += number end # Optimized iteration using `reduce` numbers = [1, 2, 3, 4, 5] sum = numbers.reduce(0, :+)
String Manipulation
Manipulating strings efficiently is crucial, especially when dealing with large amounts of textual data. Avoiding unnecessary string concatenation, using interpolation instead of string concatenation, and leveraging methods like gsub and split can help optimize string operations. Let’s consider an example:
ruby # Inefficient string concatenation greeting = 'Hello, ' + name + '!' # Efficient string interpolation greeting = "Hello, #{name}!" # Inefficient splitting words = string.split(' ') # Efficient splitting words = string.split(/\s+/)
Caching and Memoization
Caching and memoization techniques can save computational resources by storing and reusing previously computed results. Ruby provides several caching mechanisms, such as Memoist and ActiveSupport::Cache, that can be easily integrated into your code. Here’s an example using memoization:
ruby require 'memoist' class Fibonacci extend Memoist def calculate(n) return n if n < 2 calculate(n - 1) + calculate(n - 2) end memoize :calculate end
Avoiding Common Pitfalls
Certain coding practices in Ruby can lead to performance issues. Avoiding excessive method calls, minimizing object allocations, and being mindful of the performance implications of using certain methods (e.g., += on strings) are essential for optimal performance. Here’s an example:
ruby # Inefficient string concatenation in a loop result = '' array.each do |item| result += item.to_s end # Efficient string concatenation using `<<` result = '' array.each do |item| result << item.to_s end
Leveraging Libraries and Tools
Ruby has a vibrant ecosystem of libraries and tools that can help optimize performance. Using libraries like fast_jsonapi for JSON serialization, fastercsv for CSV processing, and parallel for concurrent execution can provide significant performance improvements. Additionally, profiling tools like rack-mini-profiler and request-level caching libraries like Dalli can help identify and address bottlenecks in web applications.
Conclusion
Optimizing performance in Ruby is a continuous process that requires careful consideration of code design, data structures, memory management, and algorithmic complexity. By following the techniques and best practices outlined in this blog, you can significantly enhance the speed and efficiency of your Ruby applications. Remember to profile and benchmark your code to identify bottlenecks and measure improvements. With a focus on optimization, you can deliver high-performing Ruby software that meets the demands of your users and provides an exceptional user experience.
Table of Contents
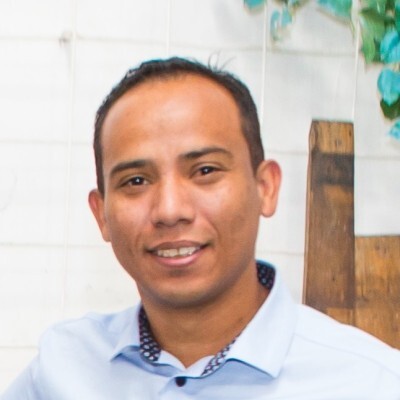
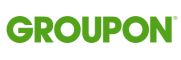