Building Recommendation Engines with Ruby: Personalized Content Suggestions
In today’s digital landscape, where the abundance of content can be overwhelming, recommendation engines play a crucial role in enhancing user engagement and satisfaction. Imagine visiting an online streaming platform, e-commerce website, or news aggregator, and being greeted with a selection of content tailored to your preferences. That’s the magic of recommendation engines. In this article, we’ll delve into the world of building personalized content suggestion systems using Ruby. We’ll explore the key concepts, algorithms, and code samples to help you get started on creating your own recommendation engine.
Table of Contents
1. Understanding Recommendation Engines
1.1. What are Recommendation Engines?
Recommendation engines, also known as recommender systems, are algorithms and techniques that analyze user data and make personalized suggestions for items or content that users might be interested in. These systems leverage historical user behavior, preferences, and sometimes contextual data to provide accurate and relevant recommendations.
1.2. Importance of Recommendation Engines
In a world flooded with content, recommendation engines help users discover new and relevant items, thus improving user engagement, increasing user retention, and driving sales in e-commerce scenarios. For businesses, these engines contribute to customer satisfaction, potentially leading to higher conversion rates and revenue.
2. Types of Recommendation Engines
There are several approaches to building recommendation engines. Here are three prominent ones:
2.1. Content-Based Filtering
Content-based filtering recommends items based on the user’s past preferences and the features of items themselves. For instance, if a user has shown interest in action movies in the past, a content-based recommendation system might suggest similar action films.
2.2. Collaborative Filtering
Collaborative filtering focuses on analyzing the interactions between users and items. It recommends items that similar users have liked or interacted with. There are two main types of collaborative filtering: user-based and item-based.
2.3. Hybrid Approaches
Hybrid approaches combine multiple recommendation techniques to improve recommendation accuracy and address the limitations of individual methods. This can lead to more robust and effective recommendation engines.
3. Building a Content-Based Recommendation Engine
A content-based recommendation engine starts by creating user profiles and analyzing the features of items. Here’s how to build one:
3.1. User Profiling
Begin by creating user profiles based on their historical preferences. These profiles capture the types of items users have liked or interacted with in the past.
3.2. TF-IDF (Term Frequency-Inverse Document Frequency)
TF-IDF is a text analysis technique that evaluates the importance of a word in a document relative to a collection of documents. It helps in understanding the significance of words in describing items.
3.3. Cosine Similarity
Cosine similarity measures the cosine of the angle between two non-zero vectors. In the context of recommendation engines, it quantifies the similarity between user profiles and item features.
Ruby Code Sample: Calculating Cosine Similarity
ruby def cosine_similarity(vector1, vector2) dot_product = vector1.dot(vector2) norm1 = Math.sqrt(vector1.dot(vector1)) norm2 = Math.sqrt(vector2.dot(vector2)) dot_product / (norm1 * norm2) end
3.4. Implementation with Ruby Code Samples
Let’s put it all together and implement a basic content-based recommendation engine in Ruby:
Ruby Code Sample: Building a Content-Based Recommendation Engine
ruby class ContentBasedRecommendationEngine def initialize(user_profiles, item_features) @user_profiles = user_profiles @item_features = item_features end def recommend(user_id) user_profile = @user_profiles[user_id] recommendations = [] @item_features.each do |item_id, item_vector| similarity = cosine_similarity(user_profile, item_vector) recommendations << { item_id: item_id, similarity: similarity } end recommendations.sort_by { |rec| rec[:similarity] }.reverse end end
4. Developing a Collaborative Filtering Recommendation Engine
Collaborative filtering leverages user interactions to make recommendations. Here are two common approaches:
4.1. User-Based Collaborative Filtering
User-based collaborative filtering recommends items that users similar to you have liked. It finds users with similar preferences and suggests items they have enjoyed.
4.2. Item-Based Collaborative Filtering
Item-based collaborative filtering identifies items that are similar to those you’ve liked in the past. It suggests items that are related to your previous interactions.
4.3. Matrix Factorization
Matrix factorization is another technique used in collaborative filtering. It decomposes the user-item interaction matrix into two lower-dimensional matrices, capturing latent factors that influence user preferences.
Ruby Code Sample: Matrix Factorization
ruby class MatrixFactorization def initialize(user_item_matrix, num_factors, num_iterations, learning_rate) # Initialization and setup end def train # Training process end def predict(user_id, item_id) # Prediction end end
5. Creating Hybrid Recommendation Engines
Hybrid recommendation engines combine content-based and collaborative filtering methods. Here’s how you can create one:
5.1. Combining Content-Based and Collaborative Filtering
Merge the recommendations from content-based and collaborative filtering approaches. Weighted combinations can be used to balance the two recommendation sources.
5.2. Weighted Hybrid Approaches
Assign different weights to content-based and collaborative filtering recommendations based on their performance or relevance.
6. Data Collection and Preprocessing
Accurate recommendation engines depend on high-quality data. Here’s how to prepare your data:
6.1. Collecting User Behavior Data
Gather data on user interactions, such as items viewed, liked, purchased, or rated.
6.2. Data Cleaning and Transformation
Process and clean the data to remove noise, handle missing values, and transform it into a suitable format for recommendation algorithms.
6.3. Integrating Data with Ruby
Use libraries like ActiveRecord in Ruby on Rails to manage and query your data efficiently.
7. Evaluating Recommendation Engines
Measuring the effectiveness of recommendation engines is crucial. Here’s how:
7.1. Accuracy Metrics
Use metrics like precision, recall, and F1-score to evaluate the accuracy of your recommendations.
7.2. A/B Testing for Recommendation Systems
Conduct A/B testing to compare the performance of different recommendation algorithms and fine-tune your system.
8. Implementing Personalization and Serendipity
Enhance user satisfaction by adding personalization and serendipity to your recommendations:
8.1. Serendipitous Recommendations
Introduce an element of surprise by occasionally recommending items that are outside the user’s typical preferences.
8.2. User Feedback Loop
Incorporate user feedback to continually improve the recommendation quality and adapt to changing preferences.
9. Deployment and Scaling Considerations
Considerations for deploying and scaling recommendation engines:
9.1. Ruby on Rails Integration
Integrate your recommendation engine into your Ruby on Rails application for seamless user experience.
9.2. Handling Large Datasets
Implement techniques like distributed computing or data sharding to handle large datasets efficiently.
9.3. Scalability and Performance
Optimize your recommendation engine for scalability and performance, ensuring quick response times even with growing user bases.
Conclusion
Building recommendation engines with Ruby opens up a world of personalization and engagement possibilities for your users. Whether you’re aiming to enhance user experience on your e-commerce platform or provide tailored content suggestions on your blog, the concepts and techniques covered in this article can serve as a solid foundation. As you dive into the world of recommendation engines, remember that continuous improvement, user feedback, and thoughtful algorithm selection are key to creating a recommendation system that truly resonates with your audience. Happy coding and recommending!
Table of Contents
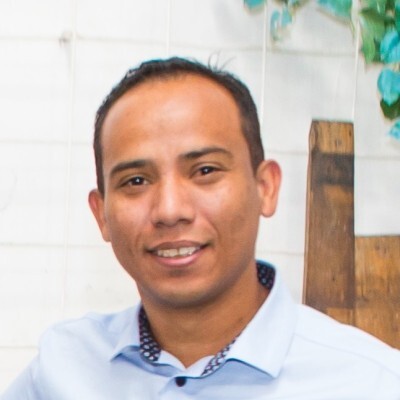
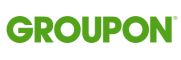