Diving into Ruby’s Regular Expressions: A Powerful Pattern Matching Tool
Regular expressions are a powerful tool for pattern matching and string manipulation. They provide a concise and flexible syntax for searching, extracting, and manipulating text data. Ruby, a dynamic and object-oriented programming language, has excellent support for regular expressions built into its core library. In this blog post, we will dive deep into Ruby’s regular expressions and explore their various features and capabilities. Whether you’re a beginner or an experienced Ruby developer, understanding regular expressions in Ruby will enhance your ability to work with text data and improve the efficiency of your code.
Basic Pattern Matching
Let’s start by looking at the basic syntax and functionality of regular expressions in Ruby. A regular expression in Ruby is defined using the forward slash (/) delimiters. For example, to match the word “ruby” in a string, we can use the following regular expression:
ruby /string/.match("I love Ruby programming!")
This will return a MatchData object containing information about the match. We can also use the =~ operator to check if a string matches a regular expression:
ruby "I love Ruby programming!" =~ /Ruby/
This will return the index of the first match or nil if no match is found.
Metacharacters and Quantifiers
Regular expressions in Ruby support a variety of metacharacters and quantifiers to define more complex patterns. Some commonly used metacharacters include:
- . (dot): Matches any single character except a newline.
- ^ (caret): Matches the beginning of a line.
- $ (dollar sign): Matches the end of a line.
- [] (square brackets): Matches any single character within the brackets.
- \ (backslash): Escapes a metacharacter to be treated as a literal character.
For example, to match any string that starts with “Hello” followed by one or more digits, we can use the following regular expression:
ruby /^Hello\d+/
Here, ^ matches the start of the line, \d matches any digit, and + indicates that the preceding character (in this case, \d) should appear one or more times.
Character Classes and Negation
Character classes allow us to define a set of characters to match against. We can use square brackets to specify a range of characters or character classes. For example, to match any lowercase vowel, we can use the following regular expression:
ruby /[aeiou]/
To match any non-vowel character, we can use the caret (^) as the first character inside the square brackets:
ruby /[^aeiou]/
This will match any character that is not a vowel.
Anchors and Word Boundaries
Anchors are used to match a pattern at a specific position within a string. The caret (^) and dollar sign ($) are examples of anchors that match the beginning and end of a line, respectively. Ruby also provides word boundaries \b to match patterns at the beginning or end of a word. For example, to match the word “ruby” as a standalone word, we can use:
ruby /\bruby\b/
This regular expression will match “ruby” but not “rubygems” or “rubymine.”
Capturing Groups and Backreferences
Capturing groups allow us to extract specific parts of a matched pattern. We can use parentheses to define a capturing group. For example, let’s say we have a string with a date in the format “DD-MM-YYYY” and we want to extract the day, month, and year separately. We can use the following regular expression:
ruby /(\d{2})-(\d{2})-(\d{4})/
By using capturing groups, we can access the matched values using the MatchData object:
ruby match_data = /(\d{2})-(\d{2})-(\d{4})/.match("Today's date is 11-07-2023") day = match_data[1] # "11" month = match_data[2] # "07" year = match_data[3] # "2023"
We can also use backreferences to refer to captured groups within the regular expression itself. For example, to match a repeated word, we can use:
ruby /\b(\w+)\s+\b/
This regular expression will match words like “hello hello” or “ruby ruby.”
<br />
Greedy and Lazy Matching
By default, regular expressions in Ruby use greedy matching, which means they try to match as much as possible. However, in some cases, we may want to perform lazy matching, where the match is made with the fewest possible characters. We can use the ? quantifier to make a quantifier lazy. For example, consider the string “1234567890”. If we want to match the smallest possible number, we can use:
ruby /\d+?/
This will match “1” instead of the whole string “1234567890”.
Conclusion
Regular expressions are a powerful tool for pattern matching and string manipulation in Ruby. They provide a concise and flexible syntax for working with text data. In this blog post, we have explored the basics of Ruby’s regular expressions, including pattern matching, metacharacters, quantifiers, character classes, anchors, capturing groups, and more. By mastering regular expressions, you can greatly enhance your ability to work with text data, improve the efficiency of your code, and unlock new possibilities in your Ruby projects. So dive in, experiment with different patterns, and unleash the full potential of Ruby’s regular expressions!
Table of Contents
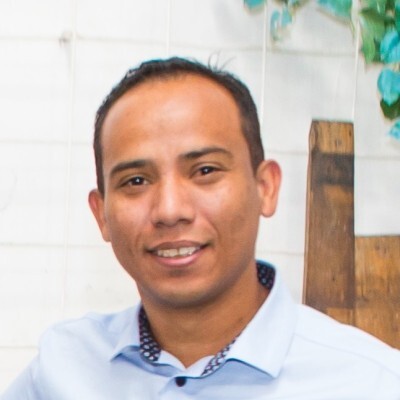
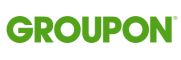