Securing Web Applications with Ruby: Vulnerabilities and Countermeasures
In the rapidly evolving digital landscape, web applications have become an integral part of our daily lives, from e-commerce platforms to social networking sites. However, this convenience comes with a significant caveat: security vulnerabilities that can expose sensitive user data, compromise user privacy, and lead to devastating consequences. Ruby is a popular programming language for developing web applications, and just like any other language, it’s crucial to understand and mitigate potential security risks. In this article, we’ll delve into the world of securing web applications built with Ruby, exploring common vulnerabilities and effective countermeasures to keep your applications and users safe.
Table of Contents
1. Understanding the Landscape
Before we delve into specific vulnerabilities and countermeasures, it’s important to have a broad understanding of the security landscape surrounding web applications. Security breaches can have severe implications, including financial losses, damage to reputation, and legal consequences. Web applications often interact with databases, external services, and user inputs, making them susceptible to various attack vectors. Here are some key points to keep in mind:
1.1. Importance of Input Validation
User inputs are a common source of vulnerabilities. Attackers can manipulate inputs to execute malicious code, such as SQL injection or cross-site scripting (XSS). Proper input validation and sanitization are essential to prevent these attacks.
1.2. Session Management and Authentication
Ensuring secure user authentication and proper session management is crucial. Weak authentication mechanisms can allow unauthorized access, while poor session management can lead to session hijacking and impersonation attacks.
1.3. Data Protection
Sensitive data, such as user passwords and personal information, must be properly encrypted both at rest and during transmission. Failure to do so can result in data leaks and breaches.
1.4. Third-Party Dependencies
Web applications often rely on third-party libraries and frameworks. Keeping these dependencies up-to-date is important, as vulnerabilities in these components can be exploited by attackers.
2. Common Vulnerabilities and Countermeasures
2.1. SQL Injection
Vulnerability: SQL injection occurs when an attacker inserts malicious SQL statements into an application’s input fields. This can lead to unauthorized access to databases and exposure of sensitive information.
Countermeasure: Use parameterized queries or prepared statements to ensure that user inputs are properly sanitized before being used in SQL queries. Here’s an example using the Ruby on Rails framework:
ruby # Vulnerable code user_input = params[:username] query = "SELECT * FROM users WHERE username = '#{user_input}'" # Countermeasure using parameterized query user_input = params[:username] query = "SELECT * FROM users WHERE username = ?" result = ActiveRecord::Base.connection.execute(query, user_input)
2.2. Cross-Site Scripting (XSS)
Vulnerability: XSS attacks involve injecting malicious scripts into a web application, which then get executed in users’ browsers. This can lead to the theft of sensitive user information or unauthorized actions on behalf of the user.
Countermeasure: Sanitize user-generated content and escape special characters before rendering them in HTML. Use security libraries like rack-attack to prevent or mitigate XSS attacks.
ruby # Vulnerable code user_input = params[:comment] render html: user_input # Countermeasure using escaping user_input = params[:comment] render html: ERB::Util.html_escape(user_input)
2.3. Cross-Site Request Forgery (CSRF)
Vulnerability: CSRF attacks trick users into performing actions they didn’t intend, often by embedding malicious requests in legitimate-looking links or forms.
Countermeasure: Use anti-CSRF tokens to validate that requests are coming from the same origin as the web application. In Ruby on Rails, you can use the form_with helper to automatically generate and validate these tokens.
ruby # Vulnerable code <form action="/change_password" method="post"> <!-- No CSRF protection --> <input type="hidden" name="new_password" value="hacker_password"> <input type="submit" value="Change Password"> </form> # Countermeasure using form_with in Rails <%= form_with(url: "/change_password", method: "post") do |form| %> <!-- Automatically includes CSRF token --> <%= form.text_field :new_password %> <%= form.submit "Change Password" %> <% end %>
2.4. Insecure Dependencies
- Vulnerability: Outdated or vulnerable third-party libraries can introduce security risks into your application.
- Countermeasure: Regularly update your dependencies to their latest secure versions. Use tools like Bundler to manage and lock dependency versions.
ruby # Gemfile gem 'rails', '6.1.4' # Specify version to avoid using the latest potentially unstable version
2.5. Insecure Direct Object References (IDOR)
- Vulnerability: IDOR occurs when an attacker can access or manipulate resources (files, URLs, records) they shouldn’t have access to.
- Countermeasure: Implement proper authorization checks to ensure that users can only access resources they’re authorized to. Use access control lists (ACLs) and well-defined roles to manage permissions.
ruby # Vulnerable code def show_profile user_id = params[:id] profile = Profile.find(user_id) render json: profile end # Countermeasure with authorization def show_profile user_id = params[:id] profile = current_user.profiles.find(user_id) render json: profile end
Conclusion
Securing web applications built with Ruby is a multifaceted challenge, but with a solid understanding of common vulnerabilities and effective countermeasures, you can significantly reduce the risk of security breaches. By prioritizing input validation, authentication, data protection, and staying vigilant about the security of your dependencies, you’ll be better equipped to create robust and resilient web applications that provide a safe user experience. Remember, web security is an ongoing process, and staying informed about the latest security best practices is crucial to maintaining the integrity of your applications and safeguarding user data.
Table of Contents
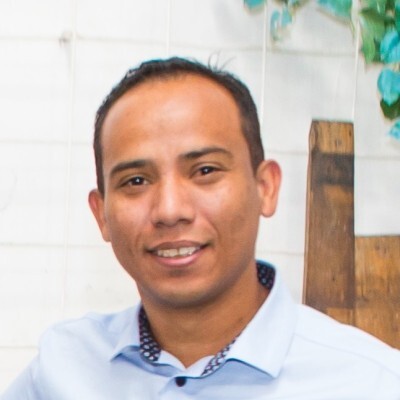
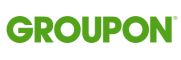