Building Sentiment Analysis Systems with Ruby: Understanding User Opinions
In the digital age, opinions and sentiments are expressed more openly and frequently than ever before. Whether it’s product reviews, social media posts, or customer feedback, understanding user opinions is crucial for businesses and organizations to make informed decisions. Sentiment analysis, also known as opinion mining, is a powerful tool that enables you to analyze and categorize text data to determine whether it expresses positive, negative, or neutral sentiments. In this blog, we will explore how to build a sentiment analysis system using the Ruby programming language, allowing you to harness the power of user opinions to your advantage.
Table of Contents
1. Introduction to Sentiment Analysis
1.1. What is Sentiment Analysis?
Sentiment analysis is the process of using natural language processing (NLP) and machine learning techniques to determine the sentiment or emotional tone of a piece of text. It classifies text as positive, negative, or neutral based on the opinions expressed within it. Sentiment analysis has a wide range of applications, from customer feedback analysis to social media monitoring and stock market prediction.
1.2. Why Sentiment Analysis Matters
Understanding user opinions is essential for businesses and organizations for several reasons:
- Customer Insights: It helps in understanding customer opinions about products and services.
- Brand Reputation: Monitoring social media sentiment can help protect and improve a brand’s reputation.
- Market Research: Analyzing sentiments in reviews and forums can provide valuable insights for market research.
- Competitor Analysis: Sentiment analysis can be used to compare the sentiment towards your products/services against competitors.
2. Setting Up Your Environment
Before we dive into building a sentiment analysis system with Ruby, you’ll need to set up your development environment. Make sure you have Ruby installed, along with any necessary gems. We’ll be using the nltk library for natural language processing, so you should have it installed as well.
ruby # Install required gems gem install nltk
3. Text Preprocessing
Text preprocessing is a crucial step in sentiment analysis. It involves cleaning and preparing the text data for analysis. Some common preprocessing steps include:
- Lowercasing: Convert all text to lowercase to ensure consistency.
- Tokenization: Split text into individual words or tokens.
- Stopword Removal: Remove common words (e.g., “and,” “the,” “is”) that don’t carry significant sentiment information.
- Stemming/Lemmatization: Reduce words to their root form (e.g., “running” becomes “run”).
Here’s an example of text preprocessing in Ruby using the nltk library:
ruby require 'nltk' def preprocess_text(text) # Lowercase the text text = text.downcase # Tokenize the text tokens = text.split # Remove stopwords stop_words = NLTK::Corpus.stopwords('english') tokens = tokens.reject { |word| stop_words.include?(word) } # Perform stemming or lemmatization stemmer = NLTK::Stem::Lancaster.new tokens = tokens.map { |word| stemmer.stem(word) } # Join the tokens back into a string preprocessed_text = tokens.join(' ') return preprocessed_text end
4. Building a Sentiment Analysis Model
Now that we have preprocessed our text data, it’s time to build a sentiment analysis model. We’ll use a simple approach by using a pre-trained model and fine-tuning it with our dataset. For this example, we’ll use the VADER sentiment analysis tool.
ruby require 'vader' # Create a SentimentIntensityAnalyzer analyzer = SentimentIntensityAnalyzer.new # Analyze a piece of text text = "I love this product! It's amazing." sentiment_scores = analyzer.sentiment_scores(text) # Interpret the sentiment scores if sentiment_scores['compound'] >= 0.05: sentiment = 'positive' elif sentiment_scores['compound'] <= -0.05: sentiment = 'negative' else: sentiment = 'neutral' puts "Sentiment: #{sentiment}"
5. Evaluating Model Performance
To ensure the accuracy of your sentiment analysis model, you need to evaluate its performance. This typically involves using a labeled dataset (containing text samples with known sentiments) and metrics such as accuracy, precision, recall, and F1-score.
ruby require 'csv' # Load a labeled dataset data = [] CSV.foreach('labeled_data.csv') do |row| text, label = row data << { text: text, label: label } end # Evaluate the model correct = 0 total = data.length data.each do |item| text = item[:text] label = item[:label] sentiment_scores = analyzer.sentiment_scores(text) if sentiment_scores['compound'] >= 0.05 && label == 'positive' correct += 1 elsif sentiment_scores['compound'] <= -0.05 && label == 'negative' correct += 1 elsif sentiment_scores['compound'].abs < 0.05 && label == 'neutral' correct += 1 end end accuracy = correct.to_f / total puts "Accuracy: #{accuracy}"
6. Deploying Your Sentiment Analysis System
Once you have a functional sentiment analysis model, you can deploy it to analyze user opinions in real-time. This can be done by integrating it into a web application, chatbot, or any other system that handles text data. Ruby provides numerous web development frameworks like Ruby on Rails and Sinatra that can help you build and deploy such applications.
Conclusion
In this blog, we explored the world of sentiment analysis and learned how to build a sentiment analysis system with Ruby. Understanding user opinions is crucial for businesses and organizations to make data-driven decisions. By preprocessing text data, building a sentiment analysis model, and evaluating its performance, you can gain valuable insights into user sentiments. Whether you want to improve customer satisfaction, monitor brand reputation, or conduct market research, sentiment analysis with Ruby can be a powerful tool in your arsenal. So, start harnessing the power of user opinions and take your decision-making to the next level with Ruby-powered sentiment analysis.
Sentiment analysis is a continuously evolving field, and there are many more advanced techniques and tools available for those who want to dive deeper. Keep exploring, experimenting, and refining your sentiment analysis system to extract even more valuable insights from user opinions. Happy coding!
Table of Contents
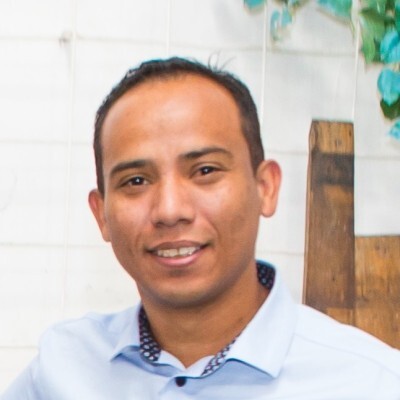
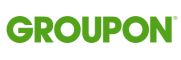