What are symbols in Ruby?
In Ruby, symbols are a unique and essential data type that serve as lightweight and immutable identifiers. Unlike strings, which are mutable and carry more overhead, symbols are often used for things like keys in hash maps, method names, and other situations where identity rather than content matters. Here’s a more detailed explanation of symbols in Ruby:
- Syntax: Symbols are represented by a colon followed by a name, like `:symbol_name`. The colon at the beginning is a syntactic indicator that distinguishes symbols from other data types.
- Immutability: Symbols are immutable, meaning their value cannot be changed once they are created. This immutability makes symbols useful in situations where you want to ensure that an identifier remains constant throughout the program’s execution.
- Memory Efficiency: Symbols are memory-efficient because they are stored in a symbol table, which essentially acts as a dictionary. When you use the same symbol multiple times in your program, Ruby reuses the same object from the symbol table, reducing memory usage. This is in contrast to strings, which create a new object every time they are used.
- Comparison Efficiency: Symbols are compared by object identity, not by content comparison like strings. This makes symbol comparisons faster because Ruby doesn’t need to check the contents of the symbols; it can simply compare their object IDs.
- Common Use Cases: Symbols are commonly used as keys in hash maps (dictionaries) because of their immutability and efficient comparison. They are also used for method names, as seen in metaprogramming and reflection.
Here’s an example of symbols in action:
```ruby # Using symbols as keys in a hash user = { :name => "John", :age => 30 } # Accessing values using symbols puts user[:name] # Outputs "John" # Symbols as method names class MyClass define_method(:my_method) do puts "Hello from my_method!" end end obj = MyClass.new obj.my_method # Calls the method defined with the symbol ```
Symbols in Ruby are lightweight, immutable identifiers used for their memory efficiency and fast comparison. They are a crucial data type, particularly in situations where identity matters more than the content of the identifier, such as hash keys and method names.
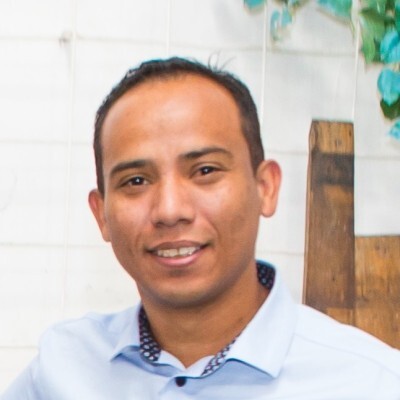
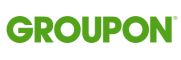