Ruby Testing Frameworks: Ensuring Code Quality and Reliability
In the world of software development, ensuring code quality and reliability is of paramount importance. Testing is a crucial aspect of the development process, as it helps identify and prevent bugs, improve code maintainability, and boost overall software quality. Ruby, a powerful and expressive programming language, provides developers with a wide range of testing frameworks to choose from. In this comprehensive guide, we will explore some of the most popular Ruby testing frameworks, their features, and best practices to ensure robust and reliable code.
Why Testing is Important in Ruby Development
Before diving into the world of Ruby testing frameworks, let’s understand why testing is essential in the development process. Testing allows developers to verify that their code functions as expected and meets the requirements set by stakeholders. Here are some key reasons why testing is important:
- Bug Detection: Testing helps identify bugs and potential issues in the codebase, allowing developers to fix them before they reach production.
- Code Maintainability: Well-tested code is easier to maintain, refactor, and extend. Tests act as documentation, providing a safety net when making changes to the codebase.
- Regression Prevention: Testing guards against regression, ensuring that new changes or features do not break existing functionality.
- Code Confidence: Robust test coverage instills confidence in developers, making it easier to deploy and iterate on the codebase.
Introduction to Ruby Testing Frameworks
Ruby has a rich ecosystem of testing frameworks that cater to various testing needs. Let’s explore some of the most popular ones:
1. Test::Unit:
Test::Unit is a testing framework bundled with Ruby and is the predecessor to MiniTest. It provides a simple and intuitive syntax for writing tests and assertions. Here’s an example of a Test::Unit test:
ruby require 'test/unit' class CalculatorTest < Test::Unit::TestCase def test_addition result = Calculator.add(2, 2) assert_equal(4, result) end end
In this example, we define a test class CalculatorTest that inherits from Test::Unit::TestCase. The test method test_addition verifies that the addition of two numbers using the Calculator class returns the expected result. The assert_equal method checks if the actual result matches the expected value.
2. RSpec:
RSpec is a widely adopted behavior-driven development (BDD) framework for Ruby. It emphasizes readability and expressive syntax, enabling developers to write tests that closely resemble natural language. Here’s an example of an RSpec test:
ruby RSpec.describe Calculator do it "returns the sum of two numbers" do result = Calculator.add(2, 2) expect(result).to eq(4) end end
In this example, we use the RSpec.describe method to define a test suite for the Calculator class. Inside the describe block, we use the it method to define a specific test case. The expect method, followed by a matcher (to eq), verifies that the actual result matches the expected value.
3. MiniTest:
MiniTest is a lightweight testing framework that ships with Ruby. It provides a minimalistic and extensible approach to testing. While it can be used as a standalone framework, it’s also the foundation for Test::Unit. Here’s an example of a MiniTest test:
ruby require 'minitest/autorun' class CalculatorTest < MiniTest::Test def test_addition result = Calculator.add(2, 2) assert_equal(4, result) end end
In this example, we define a test class CalculatorTest that inherits from MiniTest::Test. The test method test_addition asserts that the addition of two numbers using the Calculator class produces the expected result. The assert_equal method checks if the actual result matches the expected value.
4. Shoulda:
Shoulda is a testing framework built on top of Test::Unit and provides additional macros to simplify test writing. It encourages developers to write concise and readable tests. Here’s an example of a Shoulda test:
ruby require 'test/unit' require 'shoulda' class CalculatorTest < Test::Unit::TestCase should "return the sum of two numbers" do result = Calculator.add(2, 2) assert_equal(4, result) end end
In this example, we require both test/unit and shoulda libraries. Inside the test class CalculatorTest, we use the should macro to define the behavior we expect. The assert_equal method then verifies if the actual result matches the expected value.
5. Capybara:
Capybara is an acceptance testing framework that simulates user interactions with web applications. It allows developers to write expressive and powerful integration tests. Here’s an example of a Capybara test:
ruby require 'capybara/rspec' RSpec.describe "User Authentication" do it "logs in successfully" do visit "/login" fill_in "Username", with: "testuser" fill_in "Password", with: "password" click_button "Login" expect(page).to have_content("Welcome, testuser!") end end
In this example, we use Capybara’s RSpec integration to define an acceptance test for user authentication. We navigate to the login page, fill in the username and password fields, click the login button, and finally, verify that the expected welcome message is displayed on the page.
6. Cucumber:
Cucumber is a tool for behavior-driven development that enables collaboration between developers, testers, and stakeholders. It uses a plain-text syntax called Gherkin to describe application behavior. Here’s an example of a Cucumber feature:
gherkin Feature: Addition In order to verify the sum of two numbers As a developer I want to perform addition with the Calculator Scenario: Adding two numbers Given I have entered 2 into the calculator And I have entered 2 into the calculator When I press add Then the result should be 4 on the screen
In this example, we define a feature titled “Addition” using the Feature keyword. The feature describes the objective and context of the behavior being tested. Inside the feature, we define a scenario that outlines the steps to be performed. Each step uses keywords like Given, When, and Then to describe the expected behavior.
Best Practices for Ruby Testing
To ensure effective testing and maintainable test suites, it’s essential to follow best practices. Here are some guidelines to consider:
- Write Isolated Tests: Each test case should be independent of others, without relying on shared state or ordering of execution.
- Use Descriptive Test Names: Clear and descriptive test names help in understanding failures and provide documentation for the codebase.
- Aim for Small and Focused Tests: Tests should focus on testing a single behavior or concept, avoiding unnecessary complexity.
- Leverage Test Fixtures: Use fixtures or factories to create consistent and reproducible test data.
- Test All Code Paths: Ensure that tests cover different code branches, including edge cases and error scenarios.
- Practice Test-Driven Development (TDD): Write tests before implementing the functionality to guide the development process.
- Maintain Regular Test Runs: Set up automated test runs, ideally as part of a continuous integration (CI) pipeline, to catch issues early.
- Refactor Tests Alongside Code: As the codebase evolves, update and refactor tests to align with the changes.
Conclusion
Testing is an integral part of software development, and Ruby offers a plethora of testing frameworks to choose from. Understanding the features and strengths of each framework allows developers to select the most suitable one for their projects. By employing best practices and maintaining a robust test suite, developers can ensure code quality, improve reliability, and build software that meets the expectations of end-users.
In this comprehensive guide, we explored popular Ruby testing frameworks like Test::Unit, RSpec, MiniTest, Shoulda, Capybara, and Cucumber. We also discussed best practices for effective testing. Armed with this knowledge, developers can confidently employ Ruby testing frameworks to ensure code quality and reliability in their projects.
Table of Contents
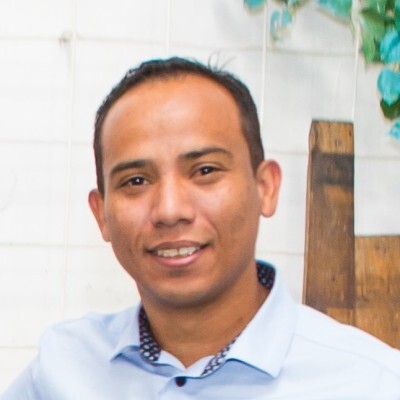
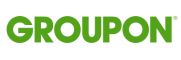