How to use the ‘unless’ statement in Ruby?
In Ruby, the `unless` statement is a conditional construct used for controlling the flow of a program. It is the opposite of the more common `if` statement and is employed when you want to execute a block of code only if a specified condition evaluates to `false`. Essentially, `unless` is a way to express “if not.”
Here’s how to use the `unless` statement in Ruby:
- Basic Usage:
You can use `unless` followed by a condition, and then specify the block of code to execute if the condition evaluates to `false`. If the condition is `true`, the code inside the `unless` block will not be executed.
```ruby unless condition # Code to execute when the condition is false end ```
For example, if you want to print a message unless a variable `x` is greater than 10:
```ruby x = 5 unless x > 10 puts "x is not greater than 10" end ```
In this case, the message will be printed because the condition `x > 10` is `false`.
- Using `else`:
You can also include an `else` clause with `unless` to specify a block of code to execute when the condition is `true`.
```ruby unless condition # Code to execute when the condition is false else # Code to execute when the condition is true end ```
Here’s an example that checks if a number is even or odd:
```ruby num = 7 unless num.even? puts "#{num} is odd" else puts "#{num} is even" end ```
Depending on whether `num` is even or odd, one of the messages will be printed.
The `unless` statement in Ruby provides an alternative way to express conditions, especially when you want to focus on what happens when a condition is not met. It can make your code more readable and expressive by emphasizing the negative condition.
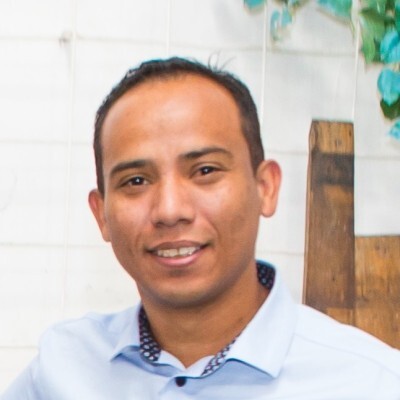
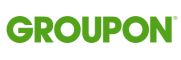