Ruby and Databases: Working with SQLite, PostgreSQL, and more
In the world of web development, databases play a vital role in storing and retrieving data efficiently. Ruby, with its elegant syntax and extensive library support, offers a seamless integration with various database systems. Whether you’re building a small application or a large-scale web platform, understanding how to work with databases in Ruby is essential.
This comprehensive guide will walk you through the process of working with two popular database systems in the Ruby ecosystem: SQLite and PostgreSQL. We’ll cover everything from setting up the databases, connecting to them using Ruby, executing queries, working with data models, and more. So let’s dive in and discover the power of Ruby and databases together!
Introduction to Databases in Ruby
Before we dive into specific databases, let’s understand the basics of working with databases in Ruby. Ruby provides several libraries and frameworks to interact with databases, such as SQLite3 and PostgreSQL. These libraries allow you to execute SQL queries, manipulate data, and handle database transactions easily.
Getting Started with SQLite
SQLite is a lightweight and embedded database engine that requires no server setup. It’s a perfect choice for small-scale applications or prototyping. In this section, we’ll cover the installation process, connecting to SQLite using Ruby, executing SQL queries, and working with data models.
1. Installing SQLite:
To work with SQLite in Ruby, we need to install the SQLite gem. Open your terminal and run the following command:
ruby gem install sqlite3
2. Connecting to SQLite with Ruby:
Connecting to an SQLite database using Ruby is straightforward. Here’s an example:
ruby require 'sqlite3' db = SQLite3::Database.new('database.db')
3. Executing SQL Queries with SQLite
SQLite supports standard SQL syntax, making it easy to execute queries. Here’s an example of executing a query and fetching results:
ruby results = db.execute('SELECT * FROM users') results.each do |row| puts row.join(', ') end
4. Working with Data Models in SQLite
To work with data models in SQLite, you can create classes representing database tables and use them to interact with the data. Here’s an example of a User class:
ruby require 'sqlite3' class User def initialize(db) @db = db end def create_table @db.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)') end def create(name) @db.execute('INSERT INTO users (name) VALUES (?)', name) end def all @db.execute('SELECT * FROM users') end end # Usage example db = SQLite3::Database.new('database.db') user = User.new(db) user.create_table user.create('John Doe') puts user.all.inspect
Exploring PostgreSQL
PostgreSQL is a powerful and feature-rich open-source relational database management system. It offers advanced functionalities and is widely used for large-scale applications. In this section, we’ll cover installing PostgreSQL, connecting to it using Ruby, executing queries, and working with data models.
1. Installing PostgreSQL
To work with PostgreSQL in Ruby, we need to install the pg gem. Open your terminal and run the following command:
ruby gem install pg
2. Connecting to PostgreSQL with Ruby
Connecting to a PostgreSQL database using Ruby requires the pg gem and the necessary connection details. Here’s an example:
ruby require 'pg' conn = PG.connect(dbname: 'mydatabase', user: 'myuser', password: 'mypassword', host: 'localhost')
3. Executing SQL Queries with PostgreSQL
Executing SQL queries with PostgreSQL in Ruby is similar to SQLite. Here’s an example:
ruby results = conn.exec('SELECT * FROM users') results.each do |row| puts row.values.join(', ') end
4. Working with Data Models in PostgreSQL
Working with data models in PostgreSQL follows a similar approach to SQLite. Here’s an example:
require 'pg' class User def initialize(conn) @conn = conn end def create_table @conn.exec('CREATE TABLE IF NOT EXISTS users (id SERIAL PRIMARY KEY, name TEXT)') end def create(name) @conn.exec_params('INSERT INTO users (name) VALUES ($1)', [name]) end def all @conn.exec('SELECT * FROM users').values end end # Usage example conn = PG.connect(dbname: 'mydatabase', user: 'myuser', password: 'mypassword', host: 'localhost') user = User.new(conn) user.create_table user.create('John Doe') puts user.all.inspect
Using ActiveRecord for Database Abstraction
ActiveRecord is an Object-Relational Mapping (ORM) library that provides an abstraction layer for working with databases in Ruby. It simplifies database operations and allows you to define models and associations. In this section, we’ll explore using ActiveRecord with SQLite and PostgreSQL.
1. Introduction to ActiveRecord
ActiveRecord is bundled with Ruby on Rails but can also be used independently. It provides a rich set of features for interacting with databases, including model definitions, query building, and data manipulation.
2. Setting up ActiveRecord with SQLite:
To use ActiveRecord with SQLite, we need to install the activerecord and sqlite3 gems. Open your terminal and run the following command:
ruby gem install activerecord sqlite3
3. Setting up ActiveRecord with PostgreSQL:
To use ActiveRecord with PostgreSQL, we need to install the pg gem and configure the database connection. Here’s an example:
ruby gem install activerecord pg
4. Migrating and Manipulating Data with ActiveRecord:
With ActiveRecord, you can define migrations to manage database schema changes and manipulate data using Ruby classes. Here’s an example of a User model:
ruby require 'active_record' class User < ActiveRecord::Base end # Usage example
ActiveRecord::Base.establish_connection(adapter: ‘sqlite3’, database: ‘database.db’)
User.connection.create_table(User.table_name) unless User.table_exists?
User.create(name: ‘John Doe’)
puts User.all.inspect
Other Database Systems
In addition to SQLite and PostgreSQL, Ruby has extensive support for other database systems. In this section, we’ll briefly touch upon working with MySQL, MongoDB, and Redis in Ruby.
1. MySQL and Ruby
To work with MySQL in Ruby, you need to install the mysql2 gem and configure the database connection. The process is similar to working with PostgreSQL, where you establish a connection and execute queries.
2. MongoDB and Ruby
For MongoDB, the mongo gem provides the necessary tools to connect, query, and manipulate data. It utilizes a NoSQL document-oriented approach and offers flexibility for schema-less data.
3. Redis and Ruby
Redis is an in-memory data structure store that can be used as a database, cache, or message broker. The redis gem allows Ruby developers to connect to Redis, store and retrieve data, and utilize its powerful features.
4. Connecting Ruby with NoSQL Databases
In addition to MongoDB and Redis, there are numerous other NoSQL databases available. Ruby offers libraries and gems for connecting and working with databases like Cassandra, CouchDB, and Neo4j, depending on your specific requirements.
Conclusion
In this comprehensive guide, we explored the power of Ruby and its integration with various databases. We covered SQLite and PostgreSQL in detail, along with an introduction to ActiveRecord for database abstraction. We also briefly touched upon working with other database systems. With this knowledge, you can confidently work with databases in your Ruby applications, leveraging the full potential of both the language and the database systems. Happy coding!
Table of Contents
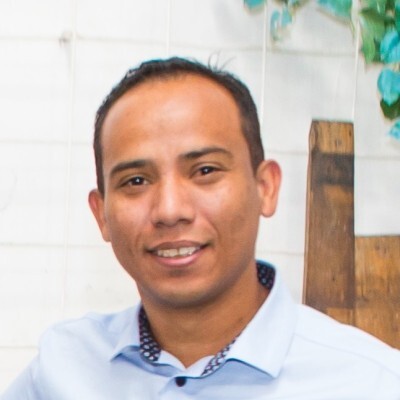
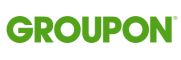