How to write a simple unit test in Ruby?
Writing simple unit tests in Ruby is a fundamental practice for ensuring the correctness of your code and maintaining its reliability as your project grows. Ruby has a built-in testing framework called MiniTest, which provides a straightforward way to write and run unit tests. Here’s a step-by-step guide on how to write a simple unit test in Ruby:
- Require the MiniTest Framework:
First, ensure that you have the MiniTest framework available in your project. If you’re using Ruby 2.2 or later, MiniTest is already included. Otherwise, you may need to install it separately.
- Create a Test File:
Conventionally, test files in Ruby are named with the suffix `_test.rb`. For example, if you have a module or class named `Calculator`, you can create a corresponding test file called `calculator_test.rb`.
- Write Test Cases:
Inside your test file, define test cases as classes that inherit from `Minitest::Test`. Each test case should include methods with names starting with `test_`, followed by a description of what the test is checking. These methods will contain your test assertions.
```ruby require 'minitest/autorun' class CalculatorTest < Minitest::Test def test_addition assert_equal 4, Calculator.add(2, 2) end def test_subtraction assert_equal 2, Calculator.subtract(4, 2) end end ```
- Use Assertions:
Inside your test methods, use MiniTest’s assertions to check whether your code produces the expected results. Common assertions include `assert_equal`, `assert`, `refute`, and more, depending on the condition you want to test.
- Run the Tests:
To execute your tests, run the `ruby` command with your test file as the argument. MiniTest will automatically discover and run your test cases, providing feedback on whether each test passed or failed.
```bash ruby calculator_test.rb ```
- Review Test Results:
MiniTest will display a summary of the test results, indicating which tests passed and which ones failed. If a test fails, you’ll receive information about the expected and actual values, helping you diagnose the issue.
By following these steps, you can write simple unit tests for your Ruby code using the MiniTest framework. This practice is essential for maintaining code quality, catching regressions, and ensuring that your code behaves as expected, especially as your project evolves.
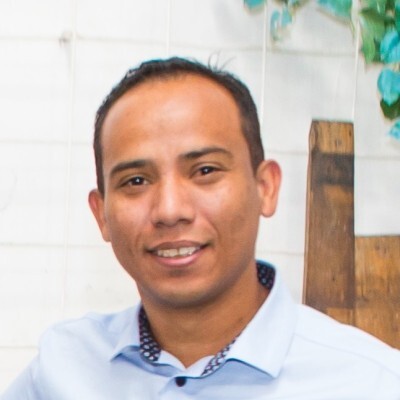
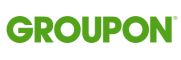