Shopify API Authentication: Securing Your Store’s Data
In the world of e-commerce, securing your store’s data is paramount. Shopify’s API allows developers to create custom applications, but with great power comes great responsibility. Ensuring that your API interactions are secure is crucial to protect sensitive data from unauthorized access. This article explores the various authentication methods provided by Shopify and how to implement them to secure your store’s data.
Understanding Shopify API Authentication
Shopify provides multiple methods for authenticating API requests, each suited for different scenarios. Whether you’re building a private app for a single store or a public app for multiple merchants, understanding and correctly implementing these authentication methods is key to maintaining data security.
1. Types of Shopify API Authentication
- Private Apps Authentication
– Used for single-store applications.
– Authentication is managed via API key and password.
Example: Basic Authentication with API Key and Password
```csharp using System; using System.Net.Http; using System.Net.Http.Headers; using System.Threading.Tasks; class Program { static async Task Main() { var client = new HttpClient(); var apiKey = "your_api_key"; var password = "your_password"; var storeUrl = "https://your-store.myshopify.com/admin/api/2023-07/products.json"; client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue( "Basic", Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes($"{apiKey}:{password}")) ); var response = await client.GetStringAsync(storeUrl); Console.WriteLine(response); } } ```
- OAuth Authentication
– Preferred for public apps.
– Utilizes OAuth 2.0 for secure, token-based authentication.
Example: OAuth 2.0 Authentication Flow
```csharp using System; using System.Net.Http; using System.Threading.Tasks; class Program { static async Task Main() { var clientId = "your_client_id"; var clientSecret = "your_client_secret"; var code = "authorization_code_provided_by_shopify"; var redirectUri = "your_redirect_uri"; var storeUrl = "https://your-store.myshopify.com/admin/oauth/access_token"; var content = new FormUrlEncodedContent(new[] { new KeyValuePair<string, string>("client_id", clientId), new KeyValuePair<string, string>("client_secret", clientSecret), new KeyValuePair<string, string>("code", code), new KeyValuePair<string, string>("redirect_uri", redirectUri) }); using (var client = new HttpClient()) { var response = await client.PostAsync(storeUrl, content); var responseString = await response.Content.ReadAsStringAsync(); Console.WriteLine(responseString); } } } ```
2. Securing API Requests
Securing API requests goes beyond just authentication. Proper use of HTTPS, rate limiting, and monitoring are essential practices to prevent abuse and ensure data integrity.
Best Practices:
- Use HTTPS: Always use HTTPS to encrypt data in transit.
- Limit Permissions: Only request the minimum permissions necessary for your app.
- Monitor Usage: Regularly monitor API usage to detect any anomalies.
3. Rotating API Keys and Secrets
Regularly rotating API keys and secrets is a good security practice to minimize the risk of unauthorized access. Shopify allows easy management of API keys and secrets from the Shopify Admin interface.
Example: Rotating an API Key
- Generate a new API key and secret from the Shopify Admin.
- Update your application to use the new credentials.
- Revoke the old API key once the new one is in use.
4. Handling API Rate Limits
Shopify imposes rate limits to prevent abuse and ensure fair usage of resources. Understanding how to handle rate limits is crucial for maintaining the reliability of your app.
Example: Handling Rate Limits
```csharp using System; using System.Net.Http; using System.Threading.Tasks; class Program { static async Task Main() { var client = new HttpClient(); var storeUrl = "https://your-store.myshopify.com/admin/api/2023-07/products.json"; try { var response = await client.GetAsync(storeUrl); if (response.Headers.Contains("X-Shopify-Shop-Api-Call-Limit")) { var rateLimitHeader = response.Headers.GetValues("X-Shopify-Shop-Api-Call-Limit").FirstOrDefault(); Console.WriteLine($"API Call Limit: {rateLimitHeader}"); } } catch (HttpRequestException e) { Console.WriteLine($"Request error: {e.Message}"); } } } ```
Conclusion
Securing your Shopify store’s data through proper API authentication is vital for maintaining the trust and safety of your e-commerce platform. By leveraging the authentication methods provided by Shopify and following best practices, you can protect your store from unauthorized access and ensure a secure environment for your customers.
Further Reading:
Table of Contents
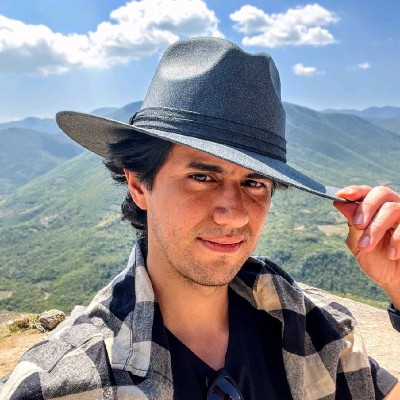
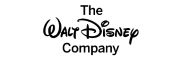