Testing Svelte Applications: Strategies and Tools for Quality Assurance
In the ever-evolving landscape of web development, creating robust and high-quality applications is paramount. Svelte, a modern JavaScript framework, has gained popularity for its efficient rendering and simplified syntax. However, delivering a flawless user experience requires thorough testing. In this blog post, we’ll delve into strategies and tools that empower you to effectively test your Svelte applications, ensuring both functionality and code reliability.
Why Testing Matters in Svelte Applications
Testing is not just a mere practice; it’s a crucial step in the development process that guarantees your application behaves as intended. Whether it’s verifying the correctness of your logic or ensuring that updates don’t break existing features, comprehensive testing can save you from unexpected issues and customer dissatisfaction.
Benefits of Testing
- Bug Detection: Testing helps catch bugs early in the development cycle, reducing the cost and effort required for bug fixes later on.
- Code Stability: Proper testing leads to stable and maintainable code, which is essential for seamless collaboration among developers.
- Enhanced User Experience: Rigorous testing ensures a smooth user experience, as users won’t encounter unexpected errors or crashes.
- Refactoring Confidence: With tests in place, you can refactor or make changes to your codebase with confidence, knowing that existing functionality won’t break.
- Regression Prevention: Regular testing prevents the reintroduction of previously fixed issues in subsequent updates.
Testing Strategies for Svelte Applications
Svelte applications can benefit from various testing strategies. Let’s explore some effective approaches to ensure the quality of your application.
1. Unit Testing
Unit testing involves testing individual units or components of your application in isolation. In the context of Svelte, this means testing components independently to ensure they work as expected.
svelte <!-- Example Svelte component --> <script> let count = 0; function increment() { count += 1; } </script> <button on:click={increment}> Click me ({count}) </button>
In this example, you could write unit tests to verify that the increment function updates the count as expected and that the button’s click event triggers the function.
2. Integration Testing
Integration testing focuses on how different components work together as a whole. In Svelte applications, this means testing the interactions between multiple components.
svelte <!-- Example Svelte components interacting --> <script> // Component A let name = "Svelte"; // Component B export let greeting; </script> <!-- Component B's usage in Component A --> <p>{greeting}, {name}!</p>
Integration tests for this scenario would ensure that when greeting is passed to Component B, it correctly displays the combined output in Component A.
3. Component Snapshot Testing
Snapshot testing captures a component’s rendered output and compares it to a saved snapshot. This ensures that the UI remains consistent over time.
javascript // Jest test for Svelte component import { render } from '@testing-library/svelte'; import MyComponent from './MyComponent.svelte'; test('MyComponent snapshot', () => { const { container } = render(MyComponent); expect(container).toMatchSnapshot(); });
4. End-to-End (E2E) Testing
End-to-end testing simulates real user scenarios by interacting with the application’s UI. Tools like Cypress or TestCafe can help you ensure that the entire application works as expected.
javascript // Cypress E2E test describe('My Svelte App', () => { it('successfully loads', () => { cy.visit('/'); cy.contains('Click me (0)').click(); cy.contains('Click me (1)'); }); });
Essential Tools for Testing Svelte Applications
To implement effective testing for your Svelte applications, you’ll need the right set of tools. Here are some essential ones to consider.
1. Jest
Jest is a widely-used testing framework that offers a simple and powerful way to write unit and integration tests for your Svelte components. It supports snapshot testing and comes with various assertion utilities.
2. Testing Library Svelte
Testing Library Svelte provides utilities to interact with and assert on Svelte components in a way that mirrors how users interact with your application.
3. Cypress
Cypress is a versatile end-to-end testing framework that allows you to write and run tests that simulate real user interactions. It provides an interactive test runner and real-time feedback.
4. TestCafe
TestCafe is another E2E testing tool that offers automated browser testing across different devices and browsers without requiring plugins.
Best Practices for Svelte Testing
While the strategies and tools provide a solid foundation, adhering to best practices ensures efficient testing. Here are some tips to optimize your Svelte testing process:
1. Keep Tests Isolated
Each test should be independent and not rely on the state or data from other tests. This prevents unexpected interactions between tests.
2. Name Tests Descriptively
Clear and descriptive test names make it easier to understand the purpose of each test, aiding collaboration among developers.
3. Use Mocks and Stubs
Use mock data and stubs to simulate external dependencies and APIs, allowing you to test components in isolation without relying on external services.
4. Prioritize UI Interactions
E2E tests should focus on critical user interactions and scenarios. Test the most common user flows to ensure a seamless user experience.
5. Regularly Update Snapshots
As your application evolves, UI changes are expected. Regularly update your snapshots to match the current UI appearance to prevent false negatives.
Conclusion
Quality assurance is an integral part of delivering exceptional user experiences through Svelte applications. By implementing the right testing strategies and leveraging powerful tools, you can confidently build and maintain applications that are reliable, stable, and feature-rich. Whether it’s unit testing, integration testing, snapshot testing, or end-to-end testing, each strategy plays a vital role in ensuring your application’s success. Remember, thorough testing not only prevents bugs but also enhances developer collaboration and user satisfaction. So, start integrating these testing practices into your Svelte development workflow and watch your application thrive in both functionality and quality.
Table of Contents
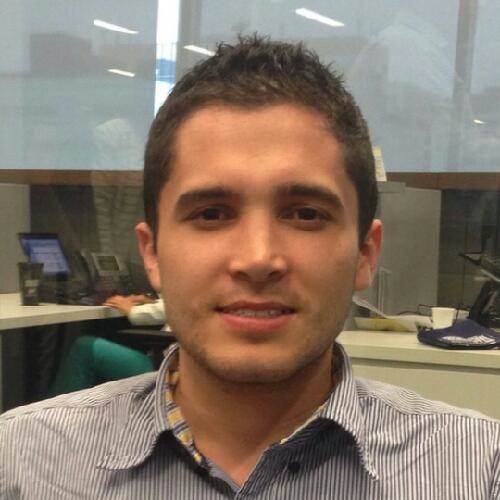
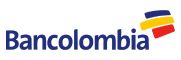