How do I use the ‘as?’ and ‘as!’ operators in Swift?
In Swift, the ‘as?’ and ‘as!’ operators are used for type casting, allowing you to work with instances of different types and ensuring type safety in your code. These operators serve distinct purposes and are essential tools in Swift’s type system.
- ‘as?’ Operator (Conditional Type Cast):
The ‘as?’ operator is used for conditional type casting. It attempts to cast an instance to a target type and returns an optional value. If the cast succeeds, it returns the instance as the target type wrapped in an Optional; otherwise, it returns nil. This operator is particularly useful when you want to handle the case where the cast might fail gracefully without causing a runtime error.
```swift let someValue: Any = 42 if let integerValue = someValue as? Int { print("The value is an integer: \(integerValue)") } else { print("The value is not an integer") } ```
In this example, the ‘as?’ operator checks if ‘someValue’ can be cast to an ‘Int’. If successful, it assigns the result to ‘integerValue’; otherwise, it gracefully handles the case where the cast fails.
- ‘as!’ Operator (Forced Type Cast):
The ‘as!’ operator, on the other hand, is used for forced type casting. It assumes that the instance can be cast to the target type and forcefully unwraps the result. If the cast fails, it triggers a runtime error. This operator should be used with caution because it doesn’t provide any safety checks and can lead to crashes if the cast is unsuccessful.
```swift let stringValue: Any = "Hello, Swift" let forcedStringValue = stringValue as! String // Use with caution, may crash if casting fails ```
In this example, ‘forcedStringValue’ is forcefully cast to ‘String’ assuming that ‘stringValue’ is of that type. However, if ‘stringValue’ were not a ‘String,’ it would result in a runtime error.
In summary, the ‘as?’ and ‘as!’ operators in Swift allow you to manage type casting in a flexible and safe way. ‘as?’ is suitable for scenarios where you want to gracefully handle potential type casting failures, while ‘as!’ should be used sparingly and with confidence in the type compatibility to avoid runtime errors.
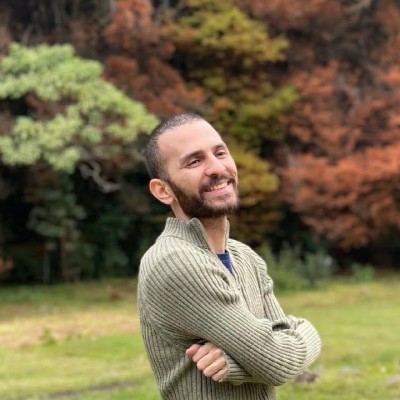
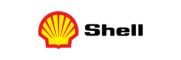