What is the ‘as’ keyword in Swift?
In Swift, the ‘as’ keyword is a fundamental part of the language used for type casting and type checking. It plays a crucial role in managing and manipulating data of different types within your code. The ‘as’ keyword primarily serves two purposes: type casting and type checking.
- Type Casting:
The ‘as’ keyword is used for type casting, which involves converting an instance of one type into another type, provided that they are related by inheritance or protocol conformance. There are two types of type casting:
– as? (Conditional Form): The ‘as?’ keyword performs a conditional type cast. It attempts to cast the instance to the target type, but if it fails (if the instance isn’t of the target type), it returns nil rather than causing a runtime error.
```swift let someValue: Any = 42 if let integerValue = someValue as? Int { print("The value is an integer: \(integerValue)") } else { print("The value is not an integer") } ```
– as! (Forced Form): The ‘as!’ keyword performs a forced type cast. It assumes that the instance can be cast to the target type, and if it fails, it triggers a runtime error if the cast isn’t possible.
```swift let stringValue: Any = "Hello, Swift" let forcedStringValue = stringValue as! String // Use with caution, may crash if casting fails ```
- Type Checking:
The ‘as’ keyword is also used for type checking, which involves determining whether an instance is of a particular type. It returns a Boolean value (true or false) based on the instance’s compatibility with the target type.
```swift if someInstance is String { print("The instance is a String") } else { print("The instance is not a String") } ```
In summary, the ‘as’ keyword in Swift is a versatile tool for working with different types of data and ensuring type safety in your code. It enables you to safely cast instances to different types and check their compatibility, reducing the risk of runtime errors and enhancing the reliability of your Swift programs.
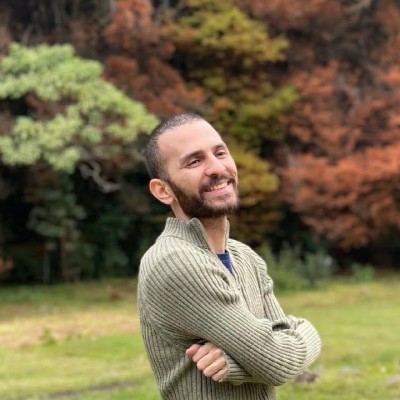
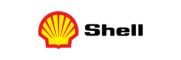