What is the ‘@autoclosure’ attribute in Swift?
The `@autoclosure` attribute in Swift is a powerful and convenient tool for simplifying code when working with closures, specifically when you want to delay the evaluation of an expression until it’s actually needed. It’s particularly useful in scenarios where you have a function or a closure that takes another closure as a parameter, and you want to defer the execution of that closure.
Here’s how `@autoclosure` works:
- Function Parameter: You apply the `@autoclosure` attribute to a function parameter that expects a closure. This closure is automatically wrapped in an anonymous closure by the Swift compiler.
- Lazy Evaluation: Instead of immediately executing the closure when the function is called, the expression inside the closure is only evaluated when it’s accessed within the function.
- Concise Syntax: This attribute provides a more concise syntax when invoking the function because you can pass the argument as if it were a regular value or expression without explicitly defining a closure.
Here’s a simple example of how `@autoclosure` is used:
```swift func greet(_ message: @autoclosure () -> String) { print("Hello, \(message())!") } // You can call greet without explicitly creating a closure: greet("world") // Output: "Hello, world!" ```
In this example, the `greet` function takes an `@autoclosure` parameter `message`. When you call `greet(“world”)`, you don’t need to wrap `”world”` in a closure explicitly. The Swift compiler handles it for you, making the code cleaner and more readable.
The primary advantage of `@autoclosure` is code readability and conciseness, especially in cases where you want to provide default values or complex expressions as arguments to functions. However, it’s essential to use it judiciously, as it can hide the fact that expensive or side-effect-producing operations are being performed, potentially leading to unexpected behavior if not used carefully.
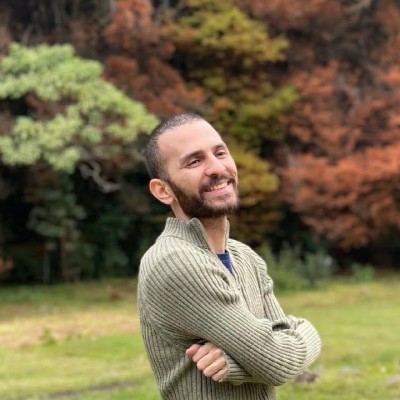
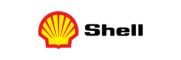