How do I use ARC (Automatic Reference Counting) in Swift?
Automatic Reference Counting (ARC) is a memory management system in Swift that automatically tracks and manages the allocation and deallocation of objects in your code. ARC ensures that objects are kept in memory as long as they are needed and are deallocated when they are no longer in use, preventing memory leaks and helping you write memory-safe code.
Here’s how you use ARC in Swift:
- Reference Counting: Every time you create a new reference to an object, ARC increases the object’s reference count by one. When references to an object are removed, ARC decreases the reference count. When the reference count reaches zero, ARC automatically deallocates the object, freeing up memory.
- Strong References: By default, Swift uses strong references, meaning that objects are kept in memory as long as there is at least one strong reference to them. You create strong references by declaring variables or properties as regular constants (let) or variables (var).
```swift class Person { var name: String init(name: String) { self.name = name } } var person1: Person? = Person(name: "Alice") // Strong reference var person2: Person? = person1 // Strong reference person1 = nil // Decrease reference count person2 = nil // Deallocate object ```
- Retain Cycles: One challenge with ARC is the potential for retain cycles, also known as strong reference cycles. This occurs when two or more objects have strong references to each other, preventing ARC from deallocating them. To avoid this, you can use weak or unowned references in Swift.
```swift class Apartment { var tenant: Person? } var alice: Person? = Person(name: "Alice") var apartment: Apartment? = Apartment() alice?.apartment = apartment // Strong reference apartment?.tenant = alice // Strong reference cycle // To break the cycle, use weak or unowned references: class Apartment { weak var tenant: Person? } ```
- Weak and Unowned References: Weak and unowned references are used when you want to prevent strong reference cycles. Weak references are typically used for optional references that can become nil, while unowned references are used when you can guarantee that the reference won’t become nil.
ARC is a fundamental and essential part of Swift’s memory management system. It takes care of memory management for you, ensuring that your objects are deallocated when they are no longer needed and helping you write more reliable and memory-efficient code. Understanding how to use strong, weak, and unowned references is crucial for avoiding retain cycles and making the most of Swift’s memory management capabilities.
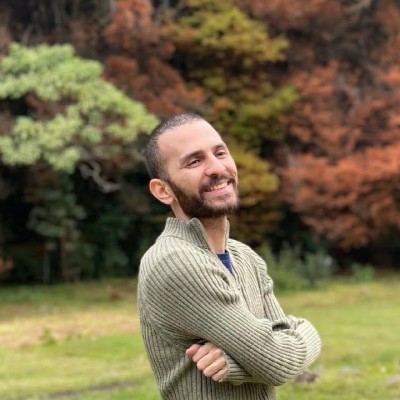
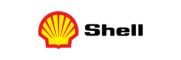