Creating Cinematic Experiences in iOS Apps: A Swift & AVFoundation Tutorial
Multimedia is an integral component of modern iOS apps. From audio playback to video streaming, interactive editing, and more, incorporating multimedia often enhances user experience. If you’re looking to optimize these functionalities, it might be time to hire Swift developers. Apple’s AVFoundation framework is the keystone for multimedia programming on iOS, offering a comprehensive suite of tools to work with audio and video. In this blog post, we will delve into how to utilize AVFoundation with Swift to manage multimedia in iOS apps, illustrated by practical examples.
Table of Contents
1. What is AVFoundation?
Before we jump into examples, let’s briefly understand AVFoundation. It’s a framework in iOS and macOS that provides tools to play, create, stream, and edit time-based audiovisual media. Its predecessor, QuickTime, although powerful, wasn’t as versatile as AVFoundation, which now offers features like real-time capture, playback, editing, and more in a modern object-oriented fashion.
2. Playing Audio with AVAudioPlayer
To kick things off, let’s see how we can play an audio file using `AVAudioPlayer`.
Example:
```swift import AVFoundation var audioPlayer: AVAudioPlayer? func playSound() { guard let url = Bundle.main.url(forResource: "sound", withExtension: "mp3") else { return } do { audioPlayer = try AVAudioPlayer(contentsOf: url, fileTypeHint: AVFileType.mp3.rawValue) audioPlayer?.play() } catch let error { print("Error playing audio: \(error.localizedDescription)") } } ```
Here, we load an MP3 file named `sound.mp3` from the app bundle and play it.
3. Playing Video with AVPlayerViewController
Playing a video involves a bit more setup since we need a user interface to display the video.
Example:
```swift import AVKit import AVFoundation func playVideo(from viewController: UIViewController) { guard let url = Bundle.main.url(forResource: "video", withExtension: "mp4") else { return } let player = AVPlayer(url: url) let playerViewController = AVPlayerViewController() playerViewController.player = player viewController.present(playerViewController, animated: true) { player.play() } } ```
With the above method, we can play a video named `video.mp4` from the app bundle.
4. Capturing Video with AVCaptureSession
`AVCaptureSession` is a versatile class that manages real-time capture activities.
Example:
```swift import AVFoundation var captureSession: AVCaptureSession? var videoPreviewLayer: AVCaptureVideoPreviewLayer? func setupCaptureSession() { captureSession = AVCaptureSession() guard let videoCaptureDevice = AVCaptureDevice.default(for: .video) else { return } do { let videoInput = try AVCaptureDeviceInput(device: videoCaptureDevice) if (captureSession?.canAddInput(videoInput) ?? false) { captureSession?.addInput(videoInput) } else { print("Could not add video input.") return } videoPreviewLayer = AVCaptureVideoPreviewLayer(session: captureSession!) videoPreviewLayer?.frame = view.layer.bounds view.layer.addSublayer(videoPreviewLayer!) captureSession?.startRunning() } catch let error { print("Error setting up capture session: \(error.localizedDescription)") } } ```
In the code above, we set up a basic video capture session and display the video feed in a layer.
5. Editing Media with AVMutableComposition
`AVMutableComposition` allows us to edit audio and video tracks, like trimming and concatenating.
Example:
```swift import AVFoundation func trimVideo(sourceURL: URL, startTime: CMTime, endTime: CMTime) -> AVMutableComposition? { let asset = AVAsset(url: sourceURL) let composition = AVMutableComposition() guard let videoTrack = asset.tracks(withMediaType: .video).first, let audioTrack = asset.tracks(withMediaType: .audio).first else { return nil } let videoCompositionTrack = composition.addMutableTrack(withMediaType: .video, preferredTrackID: kCMPersistentTrackID_Invalid) let audioCompositionTrack = composition.addMutableTrack(withMediaType: .audio, preferredTrackID: kCMPersistentTrackID_Invalid) do { try videoCompositionTrack?.insertTimeRange(CMTimeRange(start: startTime, end: endTime), of: videoTrack, at: .zero) try audioCompositionTrack?.insertTimeRange(CMTimeRange(start: startTime, end: endTime), of: audioTrack, at: .zero) } catch let error { print("Error trimming video: \(error.localizedDescription)") return nil } return composition } ```
The function above trims a video to the specified time range.
Conclusion
AVFoundation is a powerful framework that offers robust capabilities for multimedia management in iOS apps. Whether you’re building a media player, video editing app, or anything in-between, AVFoundation has the tools you need. If your project demands precision and expertise, it might be the right time to hire Swift developers. With Swift’s expressive syntax, integrating AVFoundation is a breeze, giving developers the power to create rich multimedia experiences for users. As with all technologies, make sure to refer to the official Apple documentation for the most up-to-date and comprehensive information.
Table of Contents
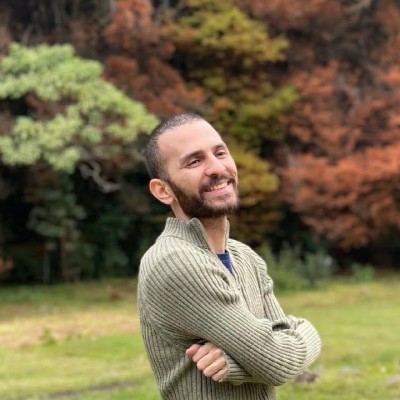
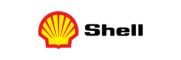