What is a ‘break’ statement in Swift?
In Swift, a ‘break’ statement is a control flow statement used to prematurely exit from a loop, switch statement, or control structure. It allows you to terminate the execution of the nearest enclosing loop or switch and continue with the code immediately after the loop or switch.
Here are some key points about the ‘break’ statement in Swift:
- Loop Termination: When used within a loop (such as ‘for-in,’ ‘while,’ or ‘repeat-while’), the ‘break’ statement allows you to exit the loop prematurely, even if the loop’s condition is still true. This is useful when you need to stop the loop based on a certain condition without waiting for the loop to complete all its iterations.
```swift for number in 1...10 { if number == 5 { break } print(number) } ```
In this example, the loop terminates when ‘number’ becomes 5, and the program continues executing the code after the loop.
- Switch Statement Termination: ‘break’ can also be used within a ‘switch’ statement to exit the switch early. It’s often used when you’ve found the case you’re interested in and want to avoid executing subsequent cases.
```swift let day = "Wednesday" switch day { case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday": print("It's a weekday.") break case "Saturday", "Sunday": print("It's a weekend.") default: print("Invalid day.") } ```
In this case, when the ‘day’ variable matches a weekday, the ‘break’ statement prevents the subsequent cases from being executed.
- Nested Loops: ‘break’ can also be used to exit from nested loops. When used within nested loops, it breaks out of the innermost loop.
```swift outerLoop: for i in 1...3 { innerLoop: for j in 1...3 { if j == 2 { break outerLoop } print("\(i), \(j)") } } ```
In this example, the ‘break’ statement with the label ‘outerLoop’ breaks out of both the inner and outer loops simultaneously.
The ‘break’ statement is a powerful tool for controlling the flow of your program, allowing you to exit loops and switch statements early when certain conditions are met, improving code efficiency and readability.
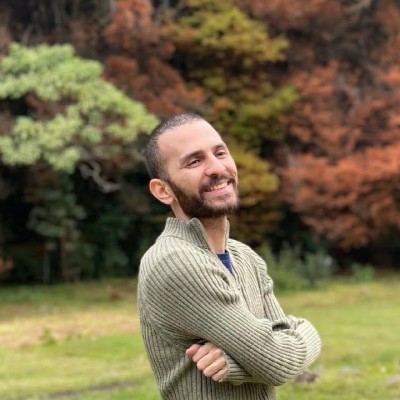
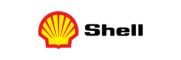