Building Dynamic User Interfaces with Swift and SwiftUI for iOS Applications
In today’s digital age, the look, feel, and functionality of your mobile application can be the defining factor that separates success from mediocrity. Particularly for iOS developers, the ability to create dynamic and engaging user interfaces (UI) using Swift can truly make your app stand out from the crowd. This is one of the reasons why many businesses opt to hire Swift iOS developers for their projects.
In this article, we’ll explore various techniques to build dynamic user interfaces with Swift, providing valuable insights for those looking to hire Swift iOS developers or improve their own Swift skills.
What is Swift?
Introduced in 2014 by Apple, Swift is a robust and intuitive programming language for iOS, macOS, watchOS, and tvOS app development. Its smooth syntax and feature-rich ecosystem have made Swift a favorite amongst developers and companies alike. Swift has a number of features that allow easy and efficient UI development such as SwiftUI, a modern way to declare user interfaces for any Apple platform.
SwiftUI for Dynamic UI
SwiftUI, an innovative, exceptionally simple way to build user interfaces across all Apple platforms with the power of Swift, has truly revolutionized UI development. This is one reason why many companies choose to hire Swift iOS developers, as they bring the capability to harness this powerful tool. SwiftUI provides views, controls, and layout structures for declaring your app’s user interface. The framework allows you to design apps in a declarative way, which is more intuitive and easier to understand than traditional imperative programming. For those seeking to hire Swift iOS developers, proficiency in SwiftUI is a key attribute to consider, given its potential to elevate your application’s UI.
Hello, SwiftUI!
For beginners, creating a simple static text label with SwiftUI can be as simple as this:
```swift import SwiftUI struct ContentView: View { var body: some View { Text("Hello, SwiftUI!") } }
The `ContentView` struct conforms to the `View` protocol and describes the view’s content and layout. `body` is a computed property that returns the description of the view.
Building a Dynamic List
One of the most common features in apps is a list that dynamically displays data. SwiftUI’s `List` can be used in combination with `ForEach` to display dynamic data. Suppose we want to display a list of names:
```swift struct ContentView: View { let names = ["Alice", "Bob", "Charlie", "Dave"] var body: some View { List(names, id: \.self) { name in Text(name) } } }
The `List` receives two arguments: an array and a closure which will return the items to be displayed on the list.
Interactive UI Elements
SwiftUI offers various interactive UI elements such as buttons, sliders, switches etc. Here is a simple example of a dynamic interactive button that changes the text on the screen when clicked:
```swift struct ContentView: View { @State private var labelText = "Click the Button" var body: some View { VStack { Text(labelText) Button("Click me") { labelText = "Button clicked!" } } } }
`@State` is a property wrapper that allows SwiftUI to re-render the `View` whenever the state changes.
Navigation and Passing Data
SwiftUI’s `NavigationView` allows easy navigation between views. Data can be passed around using `@Binding`. For instance, let’s create a list of cities, and upon clicking a city, navigate to a detail view that shows the selected city:
```swift struct ContentView: View { let cities = ["New York", "Paris", "London", "Tokyo"] var body: some View { NavigationView { List(cities, id: \.self) { city in NavigationLink(destination: DetailView(city: city)) { Text(city) } } .navigationTitle("Cities") } } } struct DetailView: View { let city: String var body: some View { Text(city) .navigationTitle(city) } }
`NavigationView` works in combination with `NavigationLink`, which takes a destination and a label as arguments.
Dynamic UI with Observable and Published
SwiftUI provides `ObservableObject` and `@Published` to handle dynamic changes in the data model. `ObservableObject` is a protocol that can be used with classes to listen to data changes. Let’s see this in action with a simple counter application:
```swift class Counter: ObservableObject { @Published var count = 0 } struct ContentView: View { @StateObject private var counter = Counter() var body: some View { VStack { Text("\(counter.count)") Button("Increment Count") { counter.count += 1 } } } }
Whenever the `count` variable changes, the view updates to reflect this thanks to the `@Published` property wrapper.
Conclusion
Swift and SwiftUI provide a powerful, intuitive way to design dynamic UI for iOS apps. With features such as SwiftUI’s declarative syntax, dynamic lists, interactive UI elements, easy navigation, and dynamic data handling with `ObservableObject` and `@Published`, building dynamic user interfaces has never been easier.
However, like all tools, SwiftUI is not a silver bullet. Depending on the project’s requirements, sometimes using UIKit may be more suitable. It’s crucial to understand both and choose the right tool for the job. This is a primary consideration for businesses looking to hire Swift iOS developers, as they must ensure that the developers understand the nuances and appropriate applications of these tools.
With the techniques we’ve covered in this guide, you’re well on your way to building dynamic, beautiful, and responsive iOS applications. Whether you’re aiming to enhance your skills or looking to hire Swift iOS developers for your project, this knowledge can be transformative. So dive in, start experimenting with Swift, and transform your UI ideas into reality!
Table of Contents
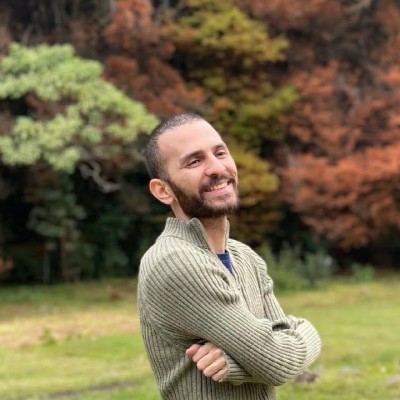
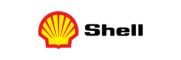