Empowering iOS App Development: A Deep Dive into the Capabilities of Swift
In the realm of iOS app development, Swift has swiftly (pun intended) ascended as a robust, versatile, and powerful language. Introduced by Apple in 2014, Swift has become an industry-favorite for its simplicity, efficiency, and performance. The growing demand to hire Swift iOS developers bears testimony to its popularity. This language is not only favored by veterans but is also remarkably accessible for beginners due to its straightforward syntax and safety features.
This post explores the power of Swift, demonstrating why businesses and developers alike are keen to hire Swift iOS developers to significantly amplify iOS app development.
Swift: The Introduction
Swift is a compiled programming language, which was primarily developed for iOS, macOS, watchOS, and tvOS app development. This language has a clear, concise syntax, and it’s considerably safer in design than Objective-C, its predecessor. Swift reduces the amount of boilerplate code, making it quicker to read, write, and modify.
To demonstrate its simplicity and power, let’s look at the classic “Hello, World!” example. Here’s how you’d write it in Swift:
```swift print("Hello, World!")
Easy, isn’t it? With one line of code, we have our first Swift program.
Swift: The Powerhouse
Swift provides developers with a wide array of powerful features that streamline the app development process. Let’s explore some key features:
Safety and Performance
Swift was designed with two main themes in mind: safety and performance. For instance, Swift uses type inference to ensure data types are correctly interpreted, reducing the likelihood of type-related errors.
Consider this:
```swift var greeting = "Hello, World!"
In the above example, we don’t explicitly declare the variable type. Swift automatically interprets it as a String, enhancing readability and speeding up the coding process.
Furthermore, Swift requires variables to be initialized before use, null pointers can’t be used, and array indices are checked for out-of-bounds errors. These are essential steps in preventing common programming errors.
Optionals
One of Swift’s strongest safety mechanisms is the Optional type. Optionals signify that a variable can hold either a value or no value (nil). It’s a great way to deal with the absence of value, which, in other languages, might lead to null-reference exceptions or similar errors.
```swift var optionalString: String? = "Hello" optionalString = nil
Interoperability with Objective-C
One of Swift’s most significant advantages is its ability to coexist with Objective-C codebases. This makes Swift an excellent choice for updating and improving legacy iOS/macOS apps. You can call Objective-C code from Swift and vice versa, making the migration process smoother.
Powerful Error Handling
Swift’s robust error handling model allows developers to catch and handle runtime errors gracefully with the help of `do-catch` blocks.
```swift do { try someFunctionThatThrows() } catch { print("An error occurred: \(error)") }
This helps make applications more resilient and reliable.
Functional Programming Patterns
Swift combines the best of procedural and object-oriented features with powerful tools that facilitate functional programming patterns. This allows you to write cleaner and more predictable code.
```swift let numbers = [1, 2, 3, 4, 5] let squaredNumbers = numbers.map { $0 * $0 } print(squaredNumbers) // prints [1, 4, 9, 16, 25]
In this example, the `map` function applies a square operation to each element in the array, showcasing how Swift employs functional programming techniques.
The Power of Swift in Real-world Applications
To truly appreciate the power of Swift, let’s discuss a couple of examples of how it’s used in
real-world applications.
Airbnb
Airbnb, one of the most popular vacation rental online marketplaces, has transitioned to Swift for their iOS app development. They’ve lauded Swift’s safety features, modern syntax, and the ease with which one can read and write Swift code. Swift’s optional handling has helped Airbnb developers write safer code and reduce the chance of unexpected crashes.
LinkedIn, a social network for professionals, also utilizes Swift for its iOS app development. The company values Swift’s type safety and ease of maintenance. Swift’s readability has also allowed LinkedIn’s development team to share and review code more effectively, speeding up the development process.
Conclusion
Swift has emerged as an industry-leading language for iOS app development due to its powerful features, safety mechanisms, and ease of use. As a result, there has been a significant rise in demand to hire Swift iOS developers. Swift’s ability to integrate with existing Objective-C codebases makes it a versatile choice for both fresh and legacy projects. From performance and safety to functional programming patterns and strong error handling, Swift empowers developers to create fast, safe, and readable code.
Whether you’re a veteran iOS developer, a business aiming to hire Swift iOS developers, or a novice looking to dive into the mobile app development world, consider Swift your go-to tool. It’s a language that truly unleashes the power of iOS app development. Swift’s journey from 2014 to the present day has shown its strength, and the increasing demand to hire Swift developers indicates a promising future.
Table of Contents
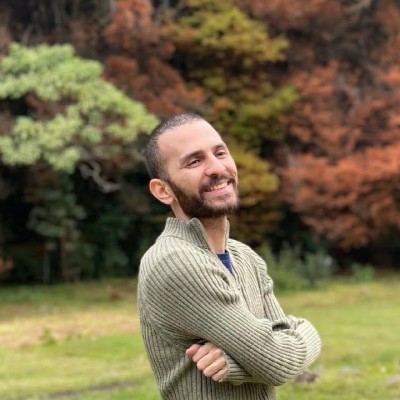
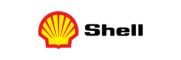