What is the ‘case let’ pattern in Swift?
In Swift, the ‘case let’ pattern is a powerful and flexible construct used within ‘switch’ statements to extract and bind associated values from enum cases. This pattern is particularly useful when dealing with enums that have associated values, allowing you to work with these values in a type-safe and expressive manner.
Here’s how the ‘case let’ pattern works:
- Matching Enum Cases with Associated Values: Enums in Swift can have associated values, which provide additional data for each enum case. When using a ‘switch’ statement to match enum cases, you can use ‘case let’ to simultaneously match a case and extract its associated value(s). For example:
```swift enum Result<T> { case success(T) case failure(Error) } let result: Result<Int> = .success(42) switch result { case let .success(value): print("Success with value: \(value)") case let .failure(error): print("Failure with error: \(error)") } ```
In this example, the ‘case let’ pattern is used to bind the associated values ‘value’ and ‘error’ to the associated values of the ‘Result’ enum case.
- Pattern Matching with Conditions: ‘case let’ can also be combined with conditions to further refine the matching criteria. For instance:
```swift let result: Result<Int> = .success(42) switch result { case let .success(value) where value > 10: print("Success with value > 10: \(value)") default: print("Other cases") } ```
Here, the ‘case let’ pattern is used to extract the associated value ‘value,’ and a condition is added to check if it’s greater than 10.
- Error Handling: ‘case let’ is commonly used in ‘switch’ statements when handling error scenarios, especially when working with Swift’s ‘Result’ type or custom error enums.
The ‘case let’ pattern enhances the expressiveness and safety of Swift code, making it easier to work with enums that encapsulate associated data. It’s a valuable tool for handling complex scenarios and ensuring that your code is both readable and robust.
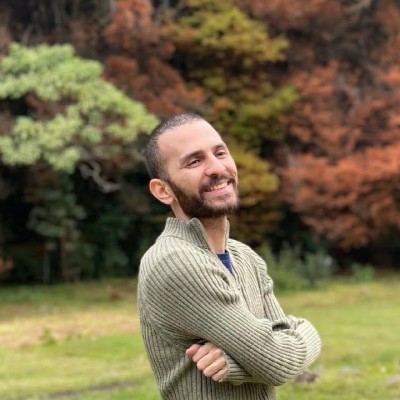
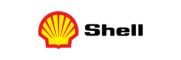