Swift and CloudKit: Syncing Data Across iOS Devices
Syncing data across multiple iOS devices is essential for providing a cohesive user experience in today’s mobile applications. CloudKit, Apple’s cloud-based framework, integrates seamlessly with Swift to facilitate data synchronization, allowing users to access their data from any device. This article explores how to use Swift and CloudKit for effective data synchronization and provides practical examples to help you get started.
Understanding CloudKit
CloudKit is a framework provided by Apple that enables developers to store and retrieve data from iCloud. It offers a robust infrastructure for syncing data across multiple devices and provides features such as data querying, public and private databases, and notifications.
Using Swift with CloudKit for Data Synchronization
Swift’s modern syntax and type safety, combined with CloudKit’s powerful features, make it an excellent choice for managing data synchronization. Below are some key aspects and code examples demonstrating how to use Swift with CloudKit to sync data across iOS devices.
1. Setting Up CloudKit
Before you can use CloudKit, you need to set up your iCloud container and configure your app to use CloudKit. Ensure you have an active Apple Developer account and that your app’s entitlements are correctly configured.
Example: Configuring CloudKit in Swift
```swift import CloudKit class CloudKitManager { private let container: CKContainer private let privateDatabase: CKDatabase init() { container = CKContainer.default() privateDatabase = container.privateCloudDatabase } // Add methods to interact with CloudKit here } ```
2. Saving Data to CloudKit
To save data to CloudKit, you’ll create `CKRecord` objects and use the `CKDatabase` methods to save them.
Example: Saving a Record
```swift import CloudKit func saveRecord() { let record = CKRecord(recordType: "Note") record["title"] = "Sample Note" as CKRecordValue record["content"] = "This is a sample note content." as CKRecordValue let database = CKContainer.default().privateCloudDatabase database.save(record) { (savedRecord, error) in if let error = error { print("Error saving record: \(error.localizedDescription)") } else { print("Record saved successfully!") } } } ```
3. Fetching Data from CloudKit
To fetch data, you’ll use queries to retrieve `CKRecord` objects from CloudKit.
Example: Fetching Records
```swift import CloudKit func fetchRecords() { let query = CKQuery(recordType: "Note", predicate: NSPredicate(value: true)) let database = CKContainer.default().privateCloudDatabase database.perform(query, inZoneWith: nil) { (records, error) in if let error = error { print("Error fetching records: \(error.localizedDescription)") } else if let records = records { for record in records { let title = record["title"] as? String ?? "No Title" let content = record["content"] as? String ?? "No Content" print("Fetched Record - Title: \(title), Content: \(content)") } } } } ```
4. Handling Record Changes
CloudKit provides notifications for changes to records, which can be used to update the UI or perform other actions in response to data changes.
Example: Observing Changes
```swift import CloudKit func observeChanges() { let subscription = CKQuerySubscription(recordType: "Note", predicate: NSPredicate(value: true), options: [.firesOnRecordCreation, .firesOnRecordUpdate]) let notificationInfo = CKSubscription.NotificationInfo() notificationInfo.alertBody = "A note has been added or updated!" notificationInfo.shouldBadge = true subscription.notificationInfo = notificationInfo let database = CKContainer.default().privateCloudDatabase database.save(subscription) { (subscription, error) in if let error = error { print("Error saving subscription: \(error.localizedDescription)") } else { print("Subscription saved successfully!") } } } ```
Conclusion
Swift and CloudKit offer a powerful combination for syncing data across iOS devices. With CloudKit’s robust infrastructure and Swift’s modern programming features, you can implement seamless data synchronization in your applications. By following the examples provided, you’ll be able to effectively manage data storage, retrieval, and synchronization, enhancing the overall user experience.
Further Reading:
Table of Contents
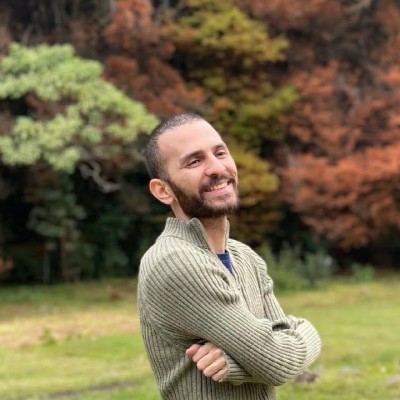
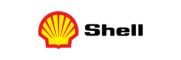