Swift and Core Data: Building Data-Driven iOS Apps
Core Data is a powerful framework for managing the model layer of an iOS application. It provides a way to manage data objects and their relationships in a robust, efficient manner. Swift, with its modern syntax and strong type system, complements Core Data by offering a seamless integration for building data-driven applications. This article explores how to utilize Swift and Core Data to handle data efficiently and build robust iOS apps.
Setting Up Core Data in a Swift Project
Before diving into code examples, you need to set up Core Data in your Swift project. When creating a new Xcode project, you can enable Core Data by checking the “Use Core Data” box. This will generate the necessary files and set up the Core Data stack for you.
Defining Your Data Model
The first step in using Core Data is defining your data model. This involves creating entities and their attributes using the Xcode data model editor.
Example: Defining a Data Model for a Task Manager App
- Create Entities: For a task manager app, you might create entities such as `Task` and `Category`.
- Define Attributes: The `Task` entity might have attributes like `title`, `dueDate`, and `isCompleted`.
Creating and Managing Core Data Entities
With your data model defined, you can now create and manage instances of your entities using Core Data.
Example: Creating and Saving a New Task
```swift import UIKit import CoreData class TaskManager { static let shared = TaskManager() private init() {} func saveTask(title: String, dueDate: Date, isCompleted: Bool) { let context = persistentContainer.viewContext let task = Task(context: context) task.title = title task.dueDate = dueDate task.isCompleted = isCompleted do { try context.save() } catch { print("Failed to save task: \(error)") } } private lazy var persistentContainer: NSPersistentContainer = { let container = NSPersistentContainer(name: "TaskModel") container.loadPersistentStores { storeDescription, error in if let error = error as NSError? { fatalError("Unresolved error \(error), \(error.userInfo)") } } return container }() } ```
Fetching and Displaying Data
Core Data allows you to fetch data from the persistent store using `NSFetchRequest`. You can use this to display data in your app.
Example: Fetching Tasks and Displaying Them
```swift import UIKit import CoreData class TaskListViewController: UITableViewController { var tasks: [Task] = [] override func viewDidLoad() { super.viewDidLoad() fetchTasks() } func fetchTasks() { let context = TaskManager.shared.persistentContainer.viewContext let fetchRequest: NSFetchRequest<Task> = Task.fetchRequest() do { tasks = try context.fetch(fetchRequest) tableView.reloadData() } catch { print("Failed to fetch tasks: \(error)") } } override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { return tasks.count } override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { let cell = tableView.dequeueReusableCell(withIdentifier: "TaskCell", for: indexPath) let task = tasks[indexPath.row] cell.textLabel?.text = task.title return cell } } ```
Updating and Deleting Data
You can also update and delete records using Core Data.
Example: Marking a Task as Completed
```swift import UIKit import CoreData class TaskManager { static let shared = TaskManager() private init() {} func markTaskAsCompleted(task: Task) { let context = persistentContainer.viewContext task.isCompleted = true do { try context.save() } catch { print("Failed to update task: \(error)") } } func deleteTask(task: Task) { let context = persistentContainer.viewContext context.delete(task) do { try context.save() } catch { print("Failed to delete task: \(error)") } } } ```
Advanced Core Data Features
Core Data also supports more advanced features such as:
– Relationships: Define relationships between entities to model complex data structures.
– Predicates: Use predicates to filter data when fetching.
– Faulting and Caching: Core Data uses faulting to efficiently manage memory and caching.
Conclusion
Swift and Core Data offer a robust solution for building data-driven iOS applications. By leveraging Core Data’s powerful data management capabilities and Swift’s modern language features, you can create efficient and scalable applications. Whether you’re managing simple data models or complex relationships, Core Data provides the tools necessary for effective data handling in your iOS apps.
Further Reading:
Table of Contents
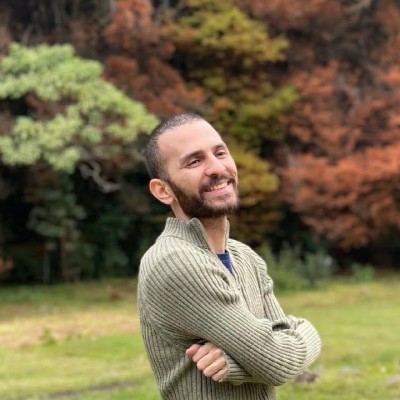
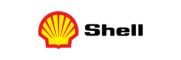