Core Data in Swift: Building Data-Driven iOS Apps
In the realm of iOS app development, handling data is a fundamental aspect that requires careful consideration. Core Data, a powerful framework provided by Apple, enables developers to efficiently manage persistent data in their applications. In this blog post, we will dive deep into the world of Core Data and explore how it can be leveraged to build data-driven iOS apps using Swift. From setting up a Core Data stack to performing complex queries, we will cover it all. So, let’s embark on this journey and unlock the full potential of Core Data.
What is Core Data?
Core Data is a powerful framework provided by Apple that allows developers to manage object graphs and persistently store data in their iOS applications. It acts as an interface between your app and the underlying data store, providing an abstraction layer that simplifies data management tasks. Core Data supports various data models, including SQLite, XML, and in-memory stores, making it a flexible choice for different app scenarios.
Setting Up Core Data Stack
Before we can start using Core Data in our iOS app, we need to set up the Core Data stack, which includes creating a data model and configuring a persistent container.
1. Creating the Data Model:
The data model defines the structure of the data we want to store. It consists of entities, attributes, and relationships. To create a data model, follow these steps:
Step 1: Open Xcode and go to File -> New -> File. Select “Data Model” under the Core Data category.
Step 2: Add entities to the data model by clicking the “+” button in the bottom-left corner of the data model editor.
Step 3: Define attributes and relationships for each entity by using the inspector pane on the right side of the editor.
2. Configuring the Persistent Container:
The persistent container is responsible for managing the interaction between our app and the data store. To configure the persistent container, add the following code to your AppDelegate.swift file:
swift lazy var persistentContainer: NSPersistentContainer = { let container = NSPersistentContainer(name: "YourDataModelName") container.loadPersistentStores(completionHandler: { (_, error) in if let error = error as NSError? { // Handle any error that occurred while loading the store. } }) return container }() func saveContext() { let context = persistentContainer.viewContext if context.hasChanges { do { try context.save() } catch { // Handle the error appropriately. } } }
Make sure to replace “YourDataModelName” with the actual name of your data model.
3. Saving and Retrieving Data:
With the Core Data stack set up, we can now save and retrieve data. To save data, create a new managed object in the context and set its properties:
php let context = persistentContainer.viewContext if let entity = NSEntityDescription.entity(forEntityName: "Person", in: context) { let person = NSManagedObject(entity: entity, insertInto: context) person.setValue("John Doe", forKey: "name") person.setValue(25, forKey: "age") do { try context.save() // Data saved successfully. } catch { // Handle the error appropriately. } }
To retrieve data, we can use a fetch request:
swift let fetchRequest: NSFetchRequest<Person> = Person.fetchRequest() do { let people = try context.fetch(fetchRequest) // Process the fetched data. } catch { // Handle the error appropriately. }
Building Relationships Between Entities
One of the key features of Core Data is its ability to establish relationships between entities. Let’s say we have two entities, “Person” and “Address,” and we want to establish a one-to-one relationship between them. Here’s how we can achieve that:
Step 1: Create an attribute in the “Person” entity of type “Address” and set the relationship type to “To One.”
Step 2: Create an inverse relationship in the “Address” entity by selecting the attribute and choosing “Inverse” in the inspector pane. Set the inverse relationship to “Person.”
Now, we can easily navigate between the “Person” and “Address” entities and fetch related data.
Fetching and Filtering Data
Core Data provides powerful features for fetching and filtering data based on specific criteria.
1. Fetching All Objects:
To fetch all objects of a particular entity, we can use a fetch request without any predicates:
swift let fetchRequest: NSFetchRequest<Person> = Person.fetchRequest() do { let people = try context.fetch(fetchRequest) // Process the fetched data. } catch { // Handle the error appropriately. }
2. Fetching Objects with Predicates:
We can use predicates to filter the fetched data based on specific conditions. For example, to fetch all people with an age greater than 30:
swift let fetchRequest: NSFetchRequest<Person> = Person.fetchRequest() fetchRequest.predicate = NSPredicate(format: "age > %@", argumentArray: [30]) do { let people = try context.fetch(fetchRequest) // Process the fetched data. } catch { // Handle the error appropriately. }
3. Sorting and Limiting Results:
We can also sort the fetched data and limit the number of results. For example, to fetch the 10 oldest people:
swift let fetchRequest: NSFetchRequest<Person> = Person.fetchRequest() fetchRequest.sortDescriptors = [NSSortDescriptor(key: "age", ascending: false)] fetchRequest.fetchLimit = 10 do { let people = try context.fetch(fetchRequest) // Process the fetched data. } catch { // Handle the error appropriately. }
Updating and Deleting Data
To update a managed object, simply modify its properties and save the context. To delete a managed object, call the delete(_:) method on the context and then save the context.
Migrating Data Models
As your app evolves, you may need to make changes to your data model. Core Data provides migration capabilities to handle these changes seamlessly. You can create a new version of the data model, set up migration mapping models, and perform lightweight or custom migrations.
Conclusion
Core Data is a powerful framework that enables developers to build data-driven iOS apps with ease. From setting up the Core Data stack to performing complex queries and managing relationships, we have explored the key aspects of Core Data in this comprehensive guide. By leveraging Core Data, you can efficiently manage persistent data in your iOS applications, providing a seamless and reliable user experience. So, dive into the world of Core Data and unlock the potential of data-driven iOS app development!
Table of Contents
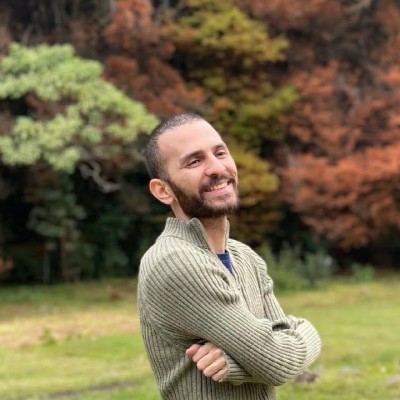
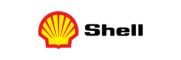