The Art of Custom Graphics: An iOS Developer’s Guide with Swift
Core Graphics is one of the foundational frameworks in iOS that provides powerful 2D drawing capabilities. While higher-level APIs like UIKit and SwiftUI often suffice for basic UI needs, sometimes, you require more fine-grained control over your graphic rendering. This is often why many companies look to hire Swift developers. In these scenarios, Core Graphics (often referred to as Quartz) shines brightly. In this blog post, we will delve deep into how Swift and Core Graphics can work harmoniously to bring your custom graphics to life in your iOS apps.
Table of Contents
1. Introduction to Core Graphics
Core Graphics is a C-based API, meaning it’s not inherently object-oriented like Swift. However, Swift’s interoperability with C makes it a seamless experience to work with Core Graphics.
At the heart of this framework is the concept of a graphics context. Think of this context as your canvas. It’s where you’ll draw paths, images, gradients, etc.
2. Drawing a Simple Shape
Let’s start by drawing a simple circle.
Steps:
- Create a new Single View App in Xcode.
- In your ViewController, override the `draw(_ rect: CGRect)` method.
```swift override func draw(_ rect: CGRect) { super.draw(rect) // Obtain graphics context guard let context = UIGraphicsGetCurrentContext() else { return } // Define circle's center and radius let center = CGPoint(x: rect.width / 2, y: rect.height / 2) let radius = min(rect.width, rect.height) / 3 // Add a circle path context.addArc(center: center, radius: radius, startAngle: 0, endAngle: 2 * .pi, clockwise: true) // Set fill color and draw path UIColor.blue.setFill() context.fillPath() } ```
Running the app now will show a blue circle in the center of the view.
3. Drawing with Paths
Paths in Core Graphics allow for more complex shapes. Let’s create a triangle.
```swift override func draw(_ rect: CGRect) { super.draw(rect) guard let context = UIGraphicsGetCurrentContext() else { return } let trianglePath = CGMutablePath() // Define triangle's vertices let vertex1 = CGPoint(x: rect.midX, y: rect.minY) let vertex2 = CGPoint(x: rect.minX, y: rect.maxY) let vertex3 = CGPoint(x: rect.maxX, y: rect.maxY) trianglePath.move(to: vertex1) trianglePath.addLine(to: vertex2) trianglePath.addLine(to: vertex3) trianglePath.closeSubpath() context.addPath(trianglePath) UIColor.green.setFill() context.fillPath() } ```
4. Drawing Gradients
Gradients can enhance UI elements with depth and a modern look. Here’s how you can draw a linear gradient:
```swift override func draw(_ rect: CGRect) { super.draw(rect) guard let context = UIGraphicsGetCurrentContext() else { return } let colorSpace = CGColorSpaceCreateDeviceRGB() // Define gradient colors let colors = [UIColor.red.cgColor, UIColor.yellow.cgColor] // Define gradient locations let locations: [CGFloat] = [0, 1] guard let gradient = CGGradient(colorsSpace: colorSpace, colors: colors as CFArray, locations: locations) else { return } // Define gradient start and end points let startPoint = CGPoint(x: rect.minX, y: rect.midY) let endPoint = CGPoint(x: rect.maxX, y: rect.midY) context.drawLinearGradient(gradient, start: startPoint, end: endPoint, options: []) } ```
5. Clipping Paths
Clipping paths let you constrain your drawing to a specific region. Let’s fill our earlier triangle with the gradient.
Incorporate the triangle drawing code and then set it as a clipping path before drawing the gradient:
```swift context.addPath(trianglePath) context.clip() // Now, add gradient drawing code here ```
The gradient will now only fill the triangle.
6. Handling Images
To draw an image:
```swift if let image = UIImage(named: "exampleImage") { image.draw(in: rect) } ```
You can also combine images with other drawing, like adding a tint to the image:
```swift context.setBlendMode(.multiply) UIColor.red.set() UIRectFillUsingBlendMode(rect, .multiply) ```
Conclusion
Core Graphics provides an arsenal of tools for drawing and manipulating graphics at a low level. The bridge between Swift and Core Graphics is smooth, enabling developers to craft intricate visuals and animations. For those businesses looking to leverage this potential, it’s often beneficial to hire Swift developers.
However, it’s crucial to remember that Core Graphics operates in a more imperative fashion, making you dictate each drawing step. While this grants you power and control, there are scenarios where higher-level UI frameworks like SwiftUI might be more suitable. Always choose the tool that aligns with your app’s requirements and your comfort level.
Embrace Core Graphics, and let your iOS apps shine with custom visuals!
Table of Contents
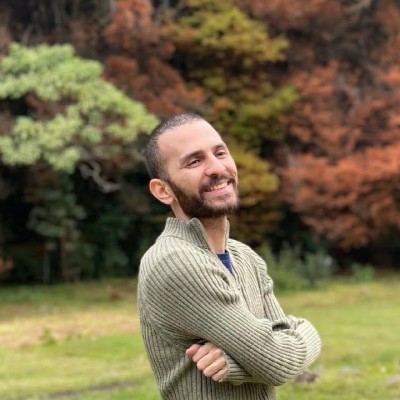
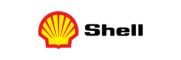