How to Drive More Engagement on iOS: Swift & Core Spotlight Unveiled
One of the most powerful features of the iOS ecosystem is Core Spotlight. With it, developers, including those looking to hire Swift developers, can make the content of their apps discoverable through the system-wide search on an iOS device. In essence, Core Spotlight lets your users search for content from your app without even opening it.
Table of Contents
In this post, we’ll explore the integration of Core Spotlight with Swift and understand how you can use it to make your app’s content more accessible to users. We’ll also see a couple of examples for clearer understanding.
1. What is Core Spotlight?
Introduced in iOS 9, Core Spotlight provides a mechanism for your app to make its content searchable through Spotlight. This is accomplished by indexing specific data in your app with Core Spotlight. When a user searches for that data on their device, your app’s content can appear in the results.
For example, consider a note-taking app. With Core Spotlight, the notes users create can be discoverable through a system-wide search, and tapping on a result would lead the user straight to the respective note inside the app.
2. Why use Core Spotlight?
- User Engagement: Even if the user forgets about an app, seeing content from it in search results can act as a reminder and potentially increase engagement.
- Convenience: Helps users find what they’re looking for quickly without navigating through multiple apps.
- Richer Experience: With deep links, you can direct the user straight to the content within your app.
3. Getting Started
To integrate Core Spotlight into your app, you’ll need to use the CoreSpotlight framework. Make sure you import it at the beginning of your Swift file:
```swift import CoreSpotlight import MobileCoreServices ```
Example 1: Indexing a Recipe App
Imagine you’ve developed a recipe app. Each recipe has a title, a description, and an image. You want these recipes to be discoverable through Spotlight search.
Here’s how you can index a recipe:
```swift func indexRecipe(recipe: Recipe) { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = recipe.title attributeSet.contentDescription = recipe.description attributeSet.thumbnailData = UIImagePNGRepresentation(recipe.image) let item = CSSearchableItem(uniqueIdentifier: "\(recipe.id)", domainIdentifier: "com.myapp.recipe", attributeSet: attributeSet) CSSearchableIndex.default().indexSearchableItems([item]) { error in if let error = error { print("Indexing error: \(error.localizedDescription)") } else { print("Recipe successfully indexed!") } } } ```
Example 2: Indexing a Book in a Reading App
For a reading app, you might want to index book titles, authors, and a brief summary. Here’s how you can do that:
```swift func indexBook(book: Book) { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = book.title attributeSet.contentDescription = "\(book.author): \(book.summary)" attributeSet.thumbnailData = UIImagePNGRepresentation(book.coverImage) let item = CSSearchableItem(uniqueIdentifier: "\(book.isbn)", domainIdentifier: "com.myapp.book", attributeSet: attributeSet) CSSearchableIndex.default().indexSearchableItems([item]) { error in if let error = error { print("Indexing error: \(error.localizedDescription)") } else { print("Book successfully indexed!") } } } ```
4. Removing Content from the Index
You can also remove content from the index when it’s no longer relevant:
```swift func deindexRecipe(recipe: Recipe) { CSSearchableIndex.default().deleteSearchableItems(withIdentifiers: ["\(recipe.id)"]) { error in if let error = error { print("Deindexing error: \(error.localizedDescription)") } else { print("Recipe successfully deindexed!") } } } ```
Conclusion
Core Spotlight provides a robust solution for making your app’s content discoverable. For businesses looking to hire Swift developers, it’s crucial to know that with just a few lines of Swift code, you can index or deindex content, enhancing the user experience manifold. Remember, the goal is not just to increase the visibility of your app but to provide a seamless user experience by helping them access your app’s content more efficiently.
Table of Contents
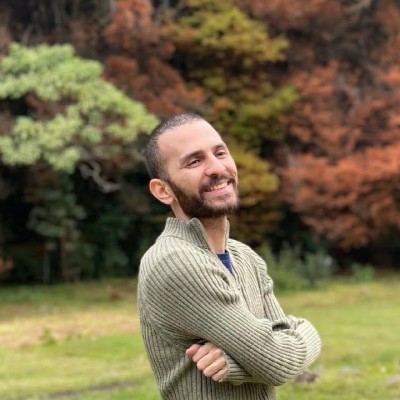
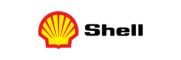