How do I create custom error types in Swift?
In Swift, creating custom error types is a fundamental aspect of robust error handling. Custom error types allow you to define specific error conditions that can occur in your code, making it easier to handle errors in a structured and meaningful way. Here’s a step-by-step guide on how to create custom error types in Swift:
- Define an Enum for Your Errors:
To create custom error types, you typically use Swift’s `enum` type. Each case of the `enum` represents a specific error condition. For example:
```swift enum NetworkError: Error { case noInternetConnection case serverError case requestTimeout } ```
In this example, we’ve defined a custom error type called `NetworkError` with three possible error cases related to network operations.
- Conform to the `Error` Protocol:
To make your `enum` usable as an error type, it should conform to the built-in `Error` protocol. Swift’s `Error` protocol doesn’t require any specific methods or properties, so you only need to declare conformance:
```swift enum NetworkError: Error { // Error cases } ```
- Throwing Custom Errors:
To indicate that a function can throw custom errors, you need to declare the `throws` keyword in the function signature and use the `throw` keyword to throw instances of your custom error type when an error condition is encountered. For example:
```swift func fetchData() throws { if !isConnectedToInternet() { throw NetworkError.noInternetConnection } // Fetch data } ```
Here, the `fetchData` function throws a `NetworkError.noInternetConnection` error if there’s no internet connection.
- Handling Custom Errors:
To handle custom errors, you use a `do-try-catch` block. In the `catch` block, you can pattern match against your custom error cases to handle them appropriately:
```swift do { try fetchData() } catch NetworkError.noInternetConnection { print("No internet connection. Please check your network.") } catch NetworkError.serverError { print("Server error. Please try again later.") } catch NetworkError.requestTimeout { print("Request timed out. Please retry the request.") } catch { print("An unknown error occurred.") } ```
In this example, we catch specific instances of `NetworkError` and provide user-friendly error messages.
Custom error types in Swift enhance the clarity and maintainability of your code by allowing you to handle errors in a structured manner. They enable you to define and communicate error conditions specific to your application domain, making it easier to write robust error-handling code.
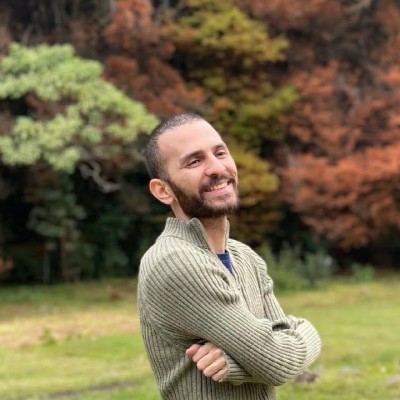
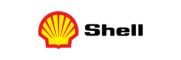