How do I create and use custom operators in Swift?
In Swift, you can create and use custom operators to extend the language’s functionality and readability of your code. Custom operators can be useful for encapsulating specific operations or enhancing the expressiveness of your code. Here’s a step-by-step guide on how to create and use custom operators in Swift:
- Operator Declaration:
To create a custom operator, you need to declare it using the `operator` keyword. Custom operators can be either prefix, infix, or postfix, and you need to specify their associativity and precedence level using the `prefix`, `infix`, or `postfix` modifiers, along with a numeric value for precedence.
```swift infix operator <^> : AdditionPrecedence ```
In this example, we’ve declared an infix custom operator `<^>` with a precedence level based on the `AdditionPrecedence`.
- Operator Implementation:
After declaring the custom operator, you need to provide its implementation using a function with the same operator symbol as the function name. For an infix operator, the function should have two parameters and return a value of the desired type.
```swift func <^> (left: Int, right: Int) -> Int { return left + (2 * right) } ```
This operator adds the left operand to twice the value of the right operand.
- Using Custom Operators:
You can now use your custom operator in your code just like built-in operators. For example:
```swift let result = 5 <^> 3 // result will be 11 ```
In this code, the custom operator `<^>` is used to perform the operation on the operands `5` and `3`, resulting in the value `11`.
- Operator Overloading:
Be mindful of operator overloading, as using custom operators excessively or with ambiguous meanings can lead to code that’s challenging to understand. It’s recommended to use custom operators sparingly and with clear intentions to improve code readability.
- Operator Precedence and Associativity:
When declaring custom operators, consider their precedence and associativity carefully to ensure they behave as expected when used alongside built-in operators.
In summary, creating and using custom operators in Swift can be a powerful way to make your code more expressive and concise, especially when you have specific operations that occur frequently in your codebase. However, it’s essential to use custom operators judiciously and follow best practices for code readability and maintainability.
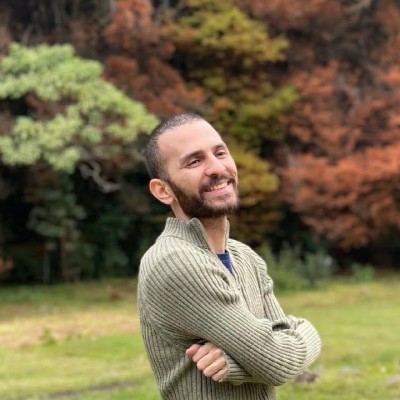
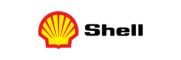