Swift Q & A
How do I create custom patterns in Swift?
Creating custom patterns in Swift allows you to extend the pattern matching capabilities of the language beyond the built-in patterns. Custom patterns are particularly useful when working with complex data structures or when you want to make your code more expressive and readable. To create custom patterns in Swift, follow these steps:
- Define a Custom Type: First, you need a custom type to work with. It can be a class, struct, enum, or any type that you want to match patterns against.
- Conform to the `Pattern` Protocol: To make your custom type matchable with patterns, you need to conform to the `Pattern` protocol. This protocol requires you to implement a single method called `match`, which takes a value of the same type as your custom pattern and returns a boolean indicating whether the pattern matches the value.
```swift struct MyCustomPattern { // Your properties and methods } extension MyCustomPattern: Pattern { func match(_ value: MyCustomPattern) -> Bool { // Implement your matching logic here return /* true if it matches, false otherwise */ } } ```
- Use Your Custom Pattern in a `case` Statement: Once you’ve defined your custom pattern and conformed to the `Pattern` protocol, you can use it in a `case` statement within a `switch` block.
```swift let valueToMatch = MyCustomPattern(/* Initialize your custom pattern */) switch valueToMatch { case MyCustomPattern(): print("Pattern matched!") default: print("Pattern not matched.") } ```
- Implement Matching Logic: Inside the `match` method, you implement the logic to compare the custom pattern against the value you want to match. Return `true` if it matches and `false` otherwise.
- Additional Customization: You can further customize your custom pattern by implementing additional methods or properties as needed for your specific use case.
Creating custom patterns in Swift can significantly enhance the readability and maintainability of your code, especially when dealing with complex data structures or domain-specific scenarios. It allows you to leverage Swift’s powerful pattern matching capabilities to express your intentions clearly and concisely.
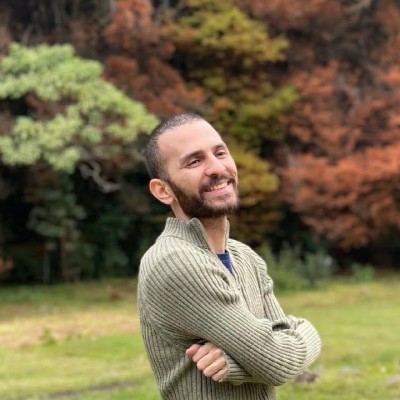
Previously at
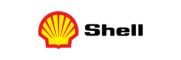
Experienced iOS Engineer with 7+ years mastering Swift. Created fintech solutions, enhanced biopharma apps, and transformed retail experiences.