How do I create and use dictionaries in Swift?
In Swift, a dictionary is a collection that stores key-value pairs. Each key in a dictionary is unique, and it’s used to access the associated value. Dictionaries are useful when you want to store and retrieve data based on a specific identifier or key. Here’s how you can create and use dictionaries:
Creating a Dictionary:
To create a dictionary in Swift, you use the `Dictionary` type, specifying the types of keys and values it will hold. You can also use Swift’s type inference to let the compiler determine the types. Here’s an example:
```swift // Explicitly specifying types var scores: Dictionary<String, Int> = [:] // Using type inference var grades = [String: String]() ```
Adding Key-Value Pairs:
You can add key-value pairs to a dictionary by assigning a value to a specific key. For example:
```swift scores["Alice"] = 95 scores["Bob"] = 89 ```
Accessing Values:
You can access the values in a dictionary using the keys. If the key exists, you’ll get the associated value; otherwise, you’ll get `nil`. For example:
```swift let aliceScore = scores["Alice"] // aliceScore is an optional Int with a value of 95 let charlieScore = scores["Charlie"] // charlieScore is nil ```
Iterating through a Dictionary:
You can iterate through the key-value pairs of a dictionary using a `for-in` loop. For example:
```swift for (name, score) in scores { print("\(name): \(score)") } ```
Modifying and Removing Key-Value Pairs:
You can change the value associated with a key or remove a key-value pair using the subscript notation or the `removeValue(forKey:)` method. For example:
```swift scores["Alice"] = 98 // Update Alice's score scores.removeValue(forKey: "Bob") // Remove Bob's score ```
Checking for Key Existence:
You can check if a key exists in a dictionary using the `contains` method or by comparing the result to `nil`. For example:
```swift if scores.keys.contains("Alice") { // Alice's score exists } if scores["Bob"] != nil { // Bob's score exists } ```
In summary, dictionaries in Swift provide a convenient way to store and retrieve data using unique keys. They are versatile data structures used in various scenarios, such as storing user information, configuration settings, and more. Swift’s dictionaries are type-safe and offer efficient key-based access, making them an essential tool for managing collections of key-value pairs in your Swift applications.
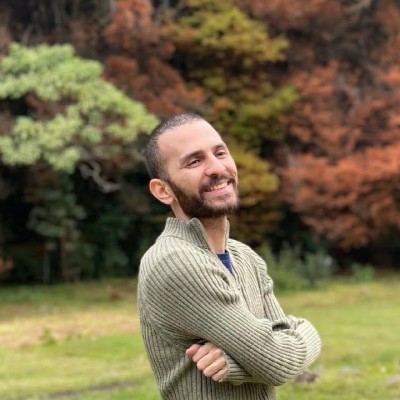
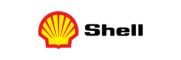