How do I use ‘enums with associated values’ in Swift?
In Swift, enums with associated values are a powerful way to define custom data types that can represent a variety of different values or states. Unlike traditional enums, which have a fixed set of cases with no associated data, enums with associated values allow you to attach additional information to each case.
Here’s how you can use enums with associated values in Swift:
- Defining an Enum with Associated Values:
To create an enum with associated values, you define the enum as usual, but you provide a placeholder for associated data within each case. For example:
```swift enum Weather { case sunny(temperature: Double) case rainy(rainfall: Double) case cloudy } ```
In this example, the `Weather` enum has three cases: `sunny`, `rainy`, and `cloudy`. The `sunny` and `rainy` cases have associated values of type `Double` to represent temperature and rainfall, respectively.
- Using Enums with Associated Values:
You can create instances of the enum by providing the associated values when initializing:
```swift let todayWeather = Weather.sunny(temperature: 28.5) let tomorrowWeather = Weather.rainy(rainfall: 10.2) let dayAfterTomorrowWeather = Weather.cloudy ```
- Accessing Associated Values:
To access the associated values, you use a `switch` statement or pattern matching:
```swift switch todayWeather { case .sunny(let temperature): print("Today is sunny with a temperature of \(temperature)°C.") case .rainy(let rainfall): print("Today is rainy with \(rainfall) mm of rain.") case .cloudy: print("Today is cloudy.") } ```
The `switch` statement allows you to extract and work with the associated values based on the enum case.
Enums with associated values are commonly used to model situations where each enum case has different data associated with it. This makes Swift enums a versatile tool for defining complex data structures and improving type safety in your code.
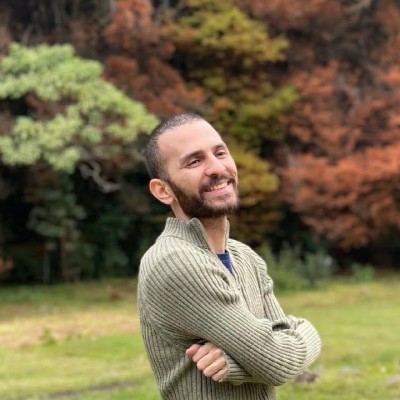
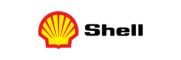