How do I use generics in Swift?
Generics in Swift are a powerful feature that allows you to write flexible and reusable code by defining functions, methods, and data structures that can work with a variety of types. Generics enable you to write code that is type-agnostic while maintaining type safety. Here’s how you can use generics in Swift:
- Defining a Generic Function or Method:
To create a generic function or method, you use angle brackets (<>) followed by a placeholder type name inside your function signature. This placeholder type can represent any data type and is typically named with a descriptive identifier. For example:
```swift func swapValues<T>(_ a: inout T, _ b: inout T) { let temp = a a = b b = temp } ```
In this example, ‘T’ is a generic type parameter that represents the type of values you want to swap.
- Using Generic Types:
You can use the generic function or method with various data types, and Swift will infer the appropriate type based on the arguments you provide:
```swift var x = 5 var y = 10 swapValues(&x, &y) // Swaps the values of 'x' and 'y' var name1 = "Alice" var name2 = "Bob" swapValues(&name1, &name2) // Swaps the values of 'name1' and 'name2' ```
- Generic Data Structures:
You can also create generic data structures like arrays, dictionaries, and stacks. Swift’s standard library provides generic collections that can work with any data type:
```swift var intArray: [Int] = [1, 2, 3] var stringArray: [String] = ["apple", "banana", "cherry"] ```
- Type Constraints:
Sometimes, you may want to impose constraints on generic types to ensure they conform to specific protocols or have certain capabilities. Swift allows you to specify type constraints using the ‘where’ clause:
```swift func processElements<T: Equatable>(_ elements: [T], target: T) -> [T] where T: Comparable { return elements.filter { $0 > target } } ```
In this example, the ‘T’ type must conform to both ‘Equatable’ and ‘Comparable’ protocols.
Generics are a fundamental aspect of Swift’s type system that promotes code reusability and type safety. They allow you to write flexible and efficient code that can work with various data types without sacrificing compile-time type checking. Generics are widely used in Swift for building generic algorithms, data structures, and functions, making your code more versatile and adaptable.
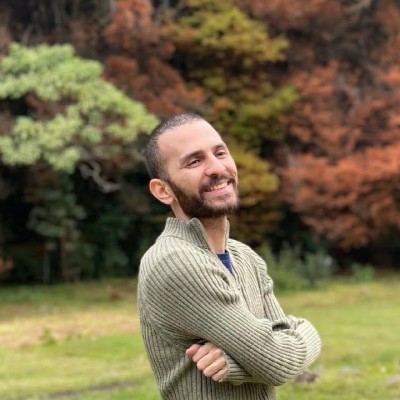
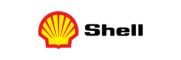