What is the ‘guard else’ statement in Swift?
The `guard-else` statement in Swift is a powerful control flow construct designed to improve code readability and safety by providing an early exit from a scope when certain conditions are not met. It’s particularly useful for handling scenarios where you want to ensure that certain conditions are true, and if they’re not, you gracefully exit the current context to avoid executing the remaining code.
Here’s how the `guard-else` statement works:
Syntax:
```swift guard condition else { // Code to execute if the condition is false // Typically includes returning, throwing, or breaking } // Code to execute if the condition is true ```
Key Points:
- Condition Check: You specify a condition that must evaluate to a Boolean value after the `guard` keyword. If the condition is `true`, execution continues normally, and there is no impact on the flow of the program.
- `else` Block: If the condition is `false`, the code within the `else` block is executed. This block typically contains code that handles the exceptional case, such as returning from a function, throwing an error, or breaking out of a loop. It’s crucial to provide a path to exit the current scope in the `else` block.
- Early Exit: One of the main benefits of `guard` is that it provides early exit from the current scope. This helps avoid deep nesting and makes the code more readable, as the normal flow of execution is at the top of the scope.
- Use Cases: `guard` is often used to validate input parameters at the beginning of a function or method, ensuring that they meet specific criteria. It’s also helpful for unwrapping optionals safely, and it’s commonly used in combination with optional binding (`if let` or `guard let`) to ensure non-nil values.
Here’s an example of how `guard` can be used to validate input parameters in a function:
```swift func processInput(age: Int?) { guard let age = age, age >= 18 else { print("Invalid age or age is below 18") return } // Code to execute when age is valid print("Welcome! You are \(age) years old.") } ```
In summary, the `guard-else` statement in Swift is a valuable tool for improving code clarity and safety by ensuring that specific conditions are met, gracefully handling exceptional cases, and providing early exits when necessary. It enhances the readability and maintainability of Swift code, making it a fundamental part of Swift development.
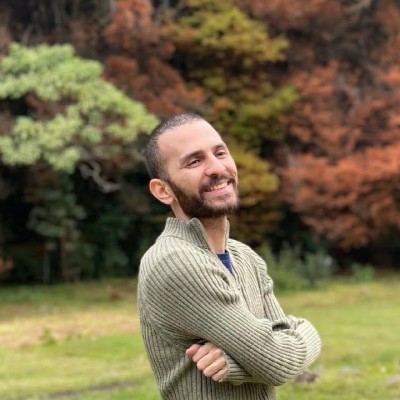
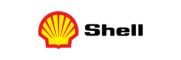