What is a guard let statement in Swift?
In Swift, a `guard let` statement is a powerful and expressive feature used for safely unwrapping optionals and performing early exits from a code block if the optional is `nil`. It’s particularly useful in scenarios where you want to ensure that an optional value exists and is not `nil` before proceeding with the execution of a function or code block. The primary purpose of `guard let` is to enhance code clarity and readability by reducing the need for nested conditional statements.
Here’s how a `guard let` statement works:
- Unwrapping Optionals: You use `guard let` to unwrap an optional value and bind it to a new non-optional variable. If the optional is `nil`, the `guard let` statement triggers an early exit from the current scope, such as a function or loop.
- Early Exit: If the `guard let` condition is not met (i.e., the optional is `nil`), the code block following the `guard let` statement is not executed. This early exit ensures that you don’t continue with potentially invalid or unsafe data.
Here’s a simple example of `guard let` in action:
```swift func processName(_ name: String?) { guard let unwrappedName = name else { print("Name is missing!") return // Exit the function if 'name' is nil } // Continue with 'unwrappedName' which is guaranteed to be non-nil print("Hello, \(unwrappedName)!") } processName("Alice") // Output: Hello, Alice! processName(nil) // Output: Name is missing! ```
In this example, the `guard let` statement safely unwraps the optional `name` and binds it to `unwrappedName`. If `name` is `nil`, it prints an error message and exits the function. If `name` contains a value, it proceeds with using `unwrappedName`.
`guard let` promotes code safety, readability, and helps prevent the dreaded “force unwrapping” of optionals, which can lead to runtime crashes. It’s widely used in Swift code to handle optionals gracefully and ensure that operations are performed only when the necessary conditions are met.
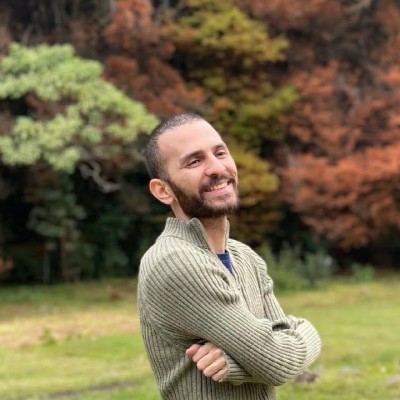
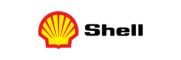