How do I handle JSON in Swift?
Handling JSON in Swift is a common task when working with APIs and data exchange in mobile and web applications. Swift provides native support for working with JSON through the `JSONDecoder` and `JSONEncoder` classes, making it straightforward to encode Swift objects into JSON and decode JSON data into Swift objects.
Here’s a step-by-step guide on how to handle JSON in Swift:
- Create Codable Structs or Classes:
Define Swift structs or classes that conform to the `Codable` protocol. This protocol combines `Encodable` (for encoding to JSON) and `Decodable` (for decoding from JSON). Each property in your struct or class should have the same name and type as the corresponding JSON keys.
```swift struct Person: Codable { var name: String var age: Int } ```
- Encoding to JSON:
To convert a Swift object into JSON data, use `JSONEncoder`:
```swift let person = Person(name: "Alice", age: 30) do { let jsonData = try JSONEncoder().encode(person) // jsonData can be sent to a server or saved to a file } catch { print("Error encoding: \(error)") } ```
- Decoding from JSON:
To parse JSON data into Swift objects, use `JSONDecoder`:
```swift let json = """ { "name": "Bob", "age": 25 } """.data(using: .utf8)! do { let decodedPerson = try JSONDecoder().decode(Person.self, from: json) print("Name: \(decodedPerson.name), Age: \(decodedPerson.age)") } catch { print("Error decoding: \(error)") } ```
- Handling Nested JSON and Arrays:
If your JSON includes nested structures or arrays, ensure that your Swift structs or classes reflect the JSON structure accordingly. Swift’s `Codable` protocol can handle nested objects and arrays seamlessly.
- Error Handling:
Always wrap JSON encoding and decoding operations in `do-catch` blocks to handle errors gracefully. JSON parsing can fail due to various reasons, such as invalid JSON format or type mismatches.
Handling JSON in Swift is made easy with the Codable protocol and the JSONEncoder and JSONDecoder classes. These tools allow you to seamlessly convert between Swift objects and JSON data, making it convenient to work with JSON APIs and data in your Swift applications.
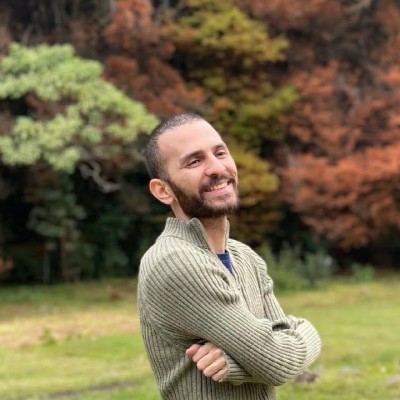
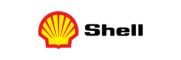