What is the difference between ‘let’ and ‘var’ in Swift?
In Swift, both ‘let’ and ‘var’ are used to declare variables, but they have significant differences in terms of mutability and value assignment.
‘let’ (Constant):
– Declaring a variable with ‘let’ creates a constant in Swift. Constants are immutable, meaning their values cannot be changed once assigned.
– You should use ‘let’ when you have a value that won’t change during the execution of your code. It’s a good practice to use constants for values that should remain constant to enhance code safety.
– Constants are often used for things like mathematical constants, configuration values, or values that shouldn’t be accidentally modified.
– Attempting to reassign a value to a constant declared with ‘let’ will result in a compilation error.
‘var’ (Variable):
– Declaring a variable with ‘var’ creates a mutable variable in Swift. Variables can have their values modified after assignment.
– You should use ‘var’ when you need a variable whose value may change during the execution of your code.
– Variables are commonly used for things like keeping track of state, accumulating values in loops, or storing data that needs to be updated over time.
– You can change the value of a variable declared with ‘var’ as many times as needed within the scope of its declaration.
Here’s a simple example to illustrate the difference:
```swift let pi = 3.14159 // 'pi' is a constant var count = 0 // 'count' is a variable count += 1 // Valid: Modifying the value of 'count' pi = 4.0 // Error: Cannot assign to 'let' constant 'pi' ```
In summary, ‘let’ is used to declare constants with immutable values, while ‘var’ is used to declare variables with mutable values. Choosing between ‘let’ and ‘var’ depends on whether you expect the value to change during the program’s execution. It’s a best practice to use ‘let’ wherever possible to ensure the immutability of values, which can lead to safer and more predictable code.
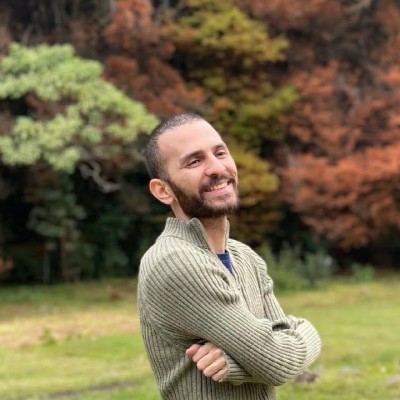
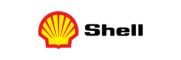