What is the ‘map’ method on optionals in Swift?
The `map` method on optionals in Swift is a powerful feature that allows you to apply a transformation to the value inside an optional if it exists. It’s a concise and safe way to work with optionals and modify their contents. Here’s a detailed explanation of how the `map` method works on optionals:
- Optional Transformation: The primary purpose of the `map` method is to apply a transformation or function to the value inside an optional if it’s not `nil`. It allows you to modify the value without explicitly unwrapping the optional. This is particularly useful when you want to chain multiple operations on optionals without introducing conditional statements.
- Syntax: The `map` method is called on an optional using the dot notation. It takes a closure as an argument, which defines the transformation to be applied to the value inside the optional. The closure receives the unwrapped value and returns a new value of the same or a different type.
- Conditional Execution: If the optional is `nil`, the `map` method simply returns `nil` without executing the closure. This behavior ensures that the transformation is only applied when there’s a valid value to work with, preventing runtime crashes.
- Chaining: One of the key benefits of the `map` method is that it can be chained with other methods or operations on optionals, such as `flatMap`, `filter`, or `compactMap`. This enables you to create a pipeline of transformations, making your code more concise and readable.
- Example Usage: Here’s a simple example of how `map` can be used to transform an optional integer:
```swift let optionalNumber: Int? = 5 let doubledNumber = optionalNumber.map { $0 * 2 } // doubledNumber is of type Int? ```
In this example, if `optionalNumber` contains a value (in this case, 5), the `map` method doubles it. If `optionalNumber` were `nil`, `doubledNumber` would also be `nil`.
- Type Consistency: The result of applying `map` is always an optional of the same type as the original optional. This ensures type consistency and helps prevent type-related errors in your code.
In summary, the `map` method on optionals in Swift provides an elegant way to safely transform the value inside an optional, keeping your code concise and readable. It promotes a functional programming style and is particularly useful when working with chains of optional operations.
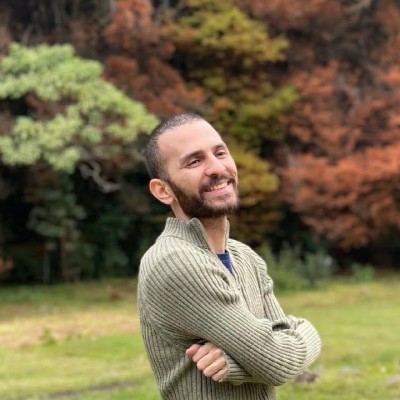
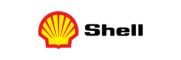