How do I work with multiple catch clauses in Swift?
Working with multiple catch clauses in Swift is essential for robust error handling in your code. Swift provides a flexible and powerful way to catch and handle different types of errors that can occur during the execution of a ‘try’ block. Multiple catch clauses allow you to specify different error cases and handle them appropriately.
Here’s how you work with multiple catch clauses in Swift:
- Using ‘do-try-catch’ Blocks:
Multiple catch clauses are typically used within a ‘do-try-catch’ block. The ‘do’ block contains the code that may throw errors, and the ‘try’ keyword is used before the code that can potentially throw an error.
- Handling Different Error Types:
You can have multiple ‘catch’ clauses, each handling a specific error type. Swift will execute the first ‘catch’ clause whose error type matches the thrown error. It’s important to order your ‘catch’ clauses from the most specific to the least specific error types.
```swift do { // Code that can throw errors } catch CustomError.errorTypeA { // Handle error of type A } catch CustomError.errorTypeB { // Handle error of type B } catch { // Handle any other errors } ```
- Custom Error Types:
It’s common practice to define custom error types using enumerations or structures to represent different error cases in your application. This allows you to provide meaningful error messages and context to the caller.
```swift enum CustomError: Error { case errorTypeA case errorTypeB } ```
- Fallback Catch Clause:
You can include a catch clause without specifying an error type at the end to handle any remaining errors that were not caught by the previous catch clauses. This is useful for handling unexpected errors or providing a generic error message.
```swift do { // Code that can throw errors } catch CustomError.errorTypeA { // Handle error of type A } catch CustomError.errorTypeB { // Handle error of type B } catch { // Handle any other errors } ```
Using multiple catch clauses in Swift allows you to create precise error handling logic tailored to different error scenarios. This ensures that your code can gracefully respond to errors and provide appropriate feedback or recovery mechanisms, improving the reliability and user experience of your applications.
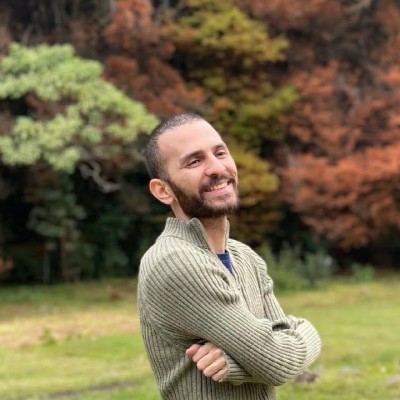
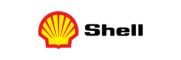