Swift Performance Optimization: Speeding Up Your iOS Apps
As an iOS app developer, one of your primary goals is to create a fast and responsive user experience. Users expect apps to load quickly, respond swiftly to interactions, and run smoothly without any glitches. Swift, Apple’s modern programming language, offers several powerful optimization techniques that can help you achieve these objectives. In this blog post, we will explore various strategies and code samples to optimize the performance of your iOS apps written in Swift.
Understanding Performance Optimization
Before diving into optimization techniques, it’s crucial to understand the importance of performance optimization and its impact on user experience. When an app performs poorly, users may get frustrated and abandon it altogether. Therefore, optimizing your app’s performance is essential to retain users and ensure their satisfaction.
Profiling and Measuring Performance
Before starting the optimization process, it’s essential to measure the performance of your app accurately. Profiling tools such as Instruments provide valuable insights into the CPU usage, memory allocation, and other performance metrics of your app. By analyzing this data, you can identify performance bottlenecks and areas that require optimization.
Reducing CPU Usage
1. Minimizing Computation Intensive Tasks
Performing heavy computations on the main thread can result in unresponsive user interfaces. To avoid this, consider moving computationally intensive tasks to background threads using GCD or Operation Queues. Here’s an example:
csharp DispatchQueue.global().async { // Perform computation-intensive task here DispatchQueue.main.async { // Update UI on the main thread } }
2. Efficient Data Structures and Algorithms
Using appropriate data structures and algorithms can significantly impact the performance of your app. Choose data structures that provide fast insertion, deletion, and retrieval operations. Additionally, leverage algorithms with optimal time complexity for common operations like sorting and searching.
Memory Management
1. Avoiding Strong Reference Cycles
Memory leaks can lead to increased memory usage, degrading your app’s performance. When working with closures or retaining objects, be mindful of strong reference cycles. Use capture lists or weak/unowned references to break reference cycles and allow objects to be deallocated when no longer needed.
2. Lazy Initialization
Lazy initialization delays the creation of an object until it’s needed, reducing memory usage and improving app startup times. Consider using lazy properties for objects that might not be accessed immediately upon app launch.
kotlin lazy var myObject: MyObject = { // Perform initialization here return MyObject() }()
Optimizing User Interface
1. Reusing Views and Cells
Reusing views and cells in table views and collection views can significantly improve scrolling performance. Utilize techniques like cell reuse identifiers and dequeueReusableCell to minimize the memory footprint and reduce the time spent creating new instances of views and cells.
2. Image Optimization
Large images can consume a significant amount of memory and impact performance. Optimize images by resizing them to the appropriate dimensions, compressing them, or using image formats that provide efficient compression, such as WebP.
Network Optimization
1. Asynchronous Network Requests
Performing network requests asynchronously prevents blocking the main thread, ensuring a responsive user interface. Use URLSession’s dataTask method with a completion handler or Combine’s network operators to execute network requests asynchronously.
2. Caching and Local Storage
Implementing caching mechanisms can reduce network requests and improve the loading speed of your app. Use URLCache or third-party libraries like AlamofireImage to cache images. Additionally, consider storing frequently accessed data locally using CoreData or Realm for faster retrieval.
Optimizing Database Operations
1. Batch Updates and Transactions
When working with databases, grouping multiple database operations into a single transaction or batch update can significantly improve performance. By reducing the number of individual disk operations, you can enhance your app’s efficiency.
2. Indexing and Query Optimization
Optimize your database queries by adding appropriate indexes to columns used in frequent search or sorting operations. Analyze and fine-tune your queries using EXPLAIN statements or database profiling tools to ensure optimal performance.
Conclusion
In this blog post, we explored various Swift optimization techniques to enhance the performance of your iOS apps. By understanding performance bottlenecks, reducing CPU usage, optimizing memory management, improving the user interface, optimizing network operations, and enhancing database operations, you can deliver a fast and responsive app to your users. Remember, continuous profiling, measuring, and refining are key to maintaining optimal performance throughout the development lifecycle.
Table of Contents
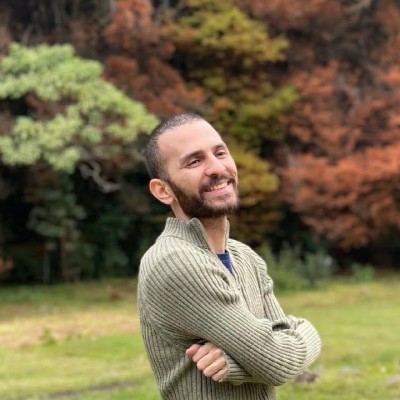
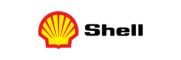