What is the ‘Self’ type in Swift?
In Swift, the `Self` type is a special type used to refer to the dynamic type of an instance within a class, structure, or enumeration. It allows you to work with the type of the current instance, making your code more flexible and adaptable. The `Self` type is particularly useful when dealing with inheritance and protocols.
Here’s a breakdown of how the `Self` type is used and its significance in Swift:
- Dynamic Type Reference:
The primary purpose of `Self` is to refer to the type of the current instance dynamically. This means that when you use `Self` in a class, structure, or enumeration, it represents the actual type of the instance, whether it’s an instance of the current type or a subclass.
```swift class Animal { func speak() { print("Animal speaks") } } class Dog: Animal { override func speak() { print("Dog barks") } func makeSound() { let myType = Self.self // Refers to the dynamic type 'Dog' print("I am a \(myType)") } } let myDog = Dog() myDog.makeSound() ```
- Protocol Requirements:
The `Self` type is commonly used in protocols to define requirements that return instances of the adopting type. It ensures that conforming types return instances of their own type, maintaining type safety.
```swift protocol Copyable { func copy() -> Self } class SomeClass: Copyable { var value: Int init(value: Int) { self.value = value } func copy() -> Self { return Self(value: self.value) } } let original = SomeClass(value: 42) let copied = original.copy() ```
In this example, `Self` ensures that the `copy` method returns an instance of the same type as the conforming class.
- Static and Class Methods:
`Self` can also be used in static and class methods to refer to the type on which they are called. This is particularly useful for creating factory methods or performing type-specific operations.
```swift class MyClass { class func createNew() -> Self { return self.init() } } let newInstance = MyClass.createNew() ```
Here, the `createNew` method returns an instance of the calling type, allowing subclasses to inherit and customize the behavior.
In summary, the `Self` type in Swift is a powerful tool for working with dynamic types, ensuring type safety in protocols, and providing flexibility in class hierarchies. It allows you to write code that adapts to the specific type of an instance at runtime, enhancing the versatility and expressiveness of your Swift programs.
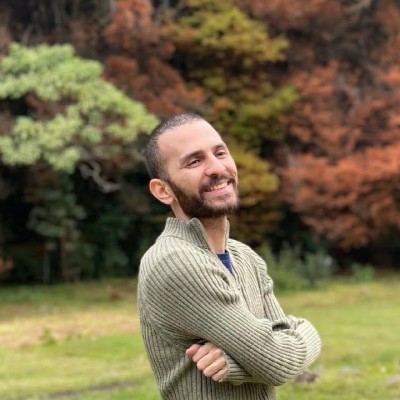
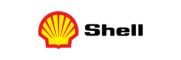