SwiftUI and Swift: Creating Beautiful and Responsive iOS Interfaces
In the ever-evolving world of iOS development, creating visually appealing and responsive interfaces is crucial to delivering exceptional user experiences. With the introduction of SwiftUI, Apple has revolutionized the way we design and build iOS apps. SwiftUI, coupled with the powerful Swift programming language, provides developers with a robust framework for crafting beautiful, intuitive, and adaptable interfaces. In this blog post, we will explore the fundamentals of SwiftUI and Swift, delve into key features, and showcase code samples to demonstrate the magic of building stunning and responsive iOS interfaces.
The Evolution of iOS Interface Development
1. The Journey from UIKit to SwiftUI
Traditionally, iOS developers relied on UIKit, a powerful framework for creating interfaces. While UIKit served its purpose well, it required developers to write a significant amount of code to achieve even the simplest of UI tasks. This led to verbose and cumbersome development workflows.
With the introduction of SwiftUI, Apple addressed these limitations by providing a declarative and intuitive way to design interfaces. SwiftUI eliminates much of the boilerplate code associated with UI development, allowing developers to focus more on the design and behavior of their app.
2. Benefits of Using SwiftUI and Swift
Using SwiftUI and Swift together offers numerous advantages. Firstly, SwiftUI enables developers to build interfaces using a declarative syntax, meaning they can describe how the UI should look and behave, and SwiftUI handles the underlying implementation details. This results in less code and increased productivity.
Additionally, SwiftUI provides real-time previews, allowing developers to see changes in their interface instantly, without the need to rebuild or rerun the app. This iterative development process significantly speeds up UI design and testing.
Furthermore, SwiftUI embraces a multiplatform approach, enabling developers to create interfaces that seamlessly adapt to various Apple platforms, such as iOS, macOS, watchOS, and even tvOS.
Understanding SwiftUI and Its Core Concepts
1. Declarative Syntax
At the heart of SwiftUI is its declarative syntax. Rather than writing imperative code to manipulate UI elements, developers describe the desired UI structure and behavior using SwiftUI’s declarative syntax. This approach allows for greater clarity and maintainability of code.
For example, to create a simple text view in SwiftUI, we can use the following code snippet:
swift struct ContentView: View { var body: some View { Text("Hello, SwiftUI!") .font(.title) .foregroundColor(.blue) } }
In the above code, we define a ContentView struct that conforms to the View protocol. We then use the Text view to display the text “Hello, SwiftUI!” with a title font and blue color.
2. View Hierarchy and Layout
In SwiftUI, views are the building blocks of user interfaces. Views can be composed hierarchically to create complex interfaces. SwiftUI takes care of laying out the views automatically, adjusting their position and size based on the available space and the defined layout rules.
Views can be nested within one another to form a view hierarchy. For instance, a button can contain a label, an image, or even another view hierarchy. This composability allows for the creation of reusable UI components.
3. State Management with Property Wrappers
State management is a critical aspect of building responsive interfaces. SwiftUI provides property wrappers like @State, @Binding, and @ObservedObject to manage and propagate changes in UI state.
@State allows a view to have mutable state that can trigger UI updates. By wrapping a property with @State, any changes to that property automatically refresh the UI.
swift struct CounterView: View { @State private var count = 0 var body: some View { VStack { Text("Count: \(count)") Button("Increment") { count += 1 } } } }
In the above example, the count property is marked with @State, enabling it to trigger UI updates whenever its value changes.
Exploring Key SwiftUI Features
1. Building Blocks: Views and Controls
SwiftUI provides a rich set of built-in views and controls, such as Text, Image, Button, and TextField. These views can be easily customized using modifiers to adjust their appearance, layout, and behavior.
swift struct ProfileView: View { var body: some View { VStack { Image("profile_picture") .resizable() .frame(width: 100, height: 100) .clipShape(Circle()) Text("John Doe") .font(.title) Button("Follow") { // Handle follow action } .padding() .background(Color.blue) .foregroundColor(.white) .cornerRadius(10) } } }
In the code above, we create a ProfileView that displays a profile picture, a name, and a follow button. We apply various modifiers to customize the appearance and behavior of the views.
2. Navigation and Presentation
SwiftUI simplifies navigation and presentation within an app by providing the NavigationView, NavigationLink, and Sheet views. These allow for seamless navigation between different views and the presentation of modal views or popovers.
swift struct ContentView: View { var body: some View { NavigationView { VStack { NavigationLink("Go to Detail", destination: DetailView()) } .navigationTitle("Home") } } } struct DetailView: View { var body: some View { Text("Detail View") .navigationTitle("Detail") } }
In the code snippet above, the NavigationView wraps the VStack containing the navigation link. When the link is tapped, the app navigates to the DetailView, which displays the text “Detail View” with a navigation title.
3. Animation and Effects
SwiftUI offers built-in support for animating and adding effects to views. Animations can be applied to individual views or to transitions between views. SwiftUI provides a wide range of animation options, including fades, rotations, scaling, and more.
swift struct AnimatedButtonView: View { @State private var isTapped = false var body: some View { Button("Tap Me") { withAnimation { isTapped.toggle() } } .padding() .background(isTapped ? Color.blue : Color.gray) .foregroundColor(.white) .cornerRadius(10) .rotationEffect(.degrees(isTapped ? 180 : 0)) } }
In the above example, when the button is tapped, the isTapped state toggles, triggering the animation. The button background color changes and the button rotates by 180 degrees.
Leveraging Swift for Enhanced Interactivity
1. Combining SwiftUI and Swift
SwiftUI seamlessly integrates with Swift, enabling developers to leverage the full power of the language for enhanced interactivity and data manipulation. With Swift, developers can implement complex algorithms, handle network requests, and perform various other tasks while still enjoying the benefits of SwiftUI’s declarative syntax.
2. Using Swift Extensions for Customization
SwiftUI supports extending existing types using Swift extensions. This allows developers to add custom functionality or modifiers to views, controls, or other SwiftUI components.
swift extension View { func roundedBorder() -> some View { self .padding() .background(Color.gray) .foregroundColor(.white) .cornerRadius(10) .overlay( RoundedRectangle(cornerRadius: 10) .stroke(Color.black, lineWidth: 1) ) } }
In the example above, we extend the View protocol to add a roundedBorder() modifier. This modifier adds a gray background, white foreground color, rounded corners, and a black border to any view that calls it.
3. Harnessing Swift’s Power for Data Manipulation
Swift provides powerful features for data manipulation, which can be used in conjunction with SwiftUI to create dynamic and data-driven interfaces. Features such as collections, optionals, functional programming concepts, and Swift’s extensive standard library can all be leveraged to manipulate and transform data within SwiftUI views.
Code Samples and Examples
1. Creating a Dynamic List View
swift struct ContentView: View { var items = ["Apple", "Banana", "Orange"] var body: some View { List(items, id: \.self) { item in Text(item) } } }
In the code above, we create a List view that displays a dynamic list of items. Each item is represented by a Text view, and the id parameter ensures proper identification and efficient updating of the list.
2. Implementing a Custom Animation
swift struct AnimatedView: View { @State private var isAnimating = false var body: some View { Rectangle() .fill(Color.blue) .frame(width: 100, height: 100) .scaleEffect(isAnimating ? 1.5 : 1.0) .animation(.spring()) .onTapGesture { withAnimation { isAnimating.toggle() } } } }
In this example, we create a Rectangle view that scales up and down when tapped. The animation is controlled by the isAnimating state, and the scaleEffect modifier adjusts the size of the rectangle based on the state value.
3. Building a Responsive Form
swift struct RegistrationView: View { @State private var name = "" @State private var email = "" var body: some View { VStack { TextField("Name", text: $name) .textFieldStyle(RoundedBorderTextFieldStyle()) .padding() TextField("Email", text: $email) .textFieldStyle(RoundedBorderTextFieldStyle()) .padding() Button("Register") { // Handle registration action } .padding() .background(Color.blue) .foregroundColor(.white) .cornerRadius(10) } .padding() } }
The above code showcases a simple registration form using SwiftUI. The @State properties name and email store the user input, and the form includes two text fields and a registration button. Modifiers are applied to customize the appearance and behavior of the views.
Best Practices for SwiftUI and Swift Development
1. Organizing and Structuring Code
As your SwiftUI project grows, it’s important to maintain a well-organized and structured codebase. Utilize SwiftUI’s view composition and embrace modularization to break down complex interfaces into reusable components. Consider adopting the Model-View-ViewModel (MVVM) architecture pattern to separate concerns and improve testability.
2. Maximizing Reusability
Leverage SwiftUI’s view composition capabilities to maximize code reuse. Encapsulate common UI patterns into reusable views or create custom modifiers and extensions to streamline repetitive tasks. Embracing reusability not only reduces code duplication but also promotes consistency and maintainability.
3. Performance Optimization Techniques
SwiftUI provides several performance optimization techniques to ensure smooth and efficient UI rendering. Utilize the @State, @Binding, and @ObservedObject property wrappers to manage UI state efficiently. Use the @ViewBuilder attribute to conditionally render views based on state, reducing unnecessary view updates. Additionally, consider using the onAppear and onDisappear modifiers to perform lazy loading and cleanup operations.
Conclusion
In this blog post, we have explored the world of SwiftUI and Swift for creating beautiful and responsive iOS interfaces. We discussed the evolution of iOS interface development, the core concepts of SwiftUI, key features, and demonstrated code samples to showcase the power and versatility of this modern framework. By harnessing the declarative syntax of SwiftUI and the expressive nature of Swift, developers can unleash their creativity and build immersive iOS experiences that captivate users. So why wait? Dive into SwiftUI and Swift, and elevate your iOS app development to new heights!
Table of Contents
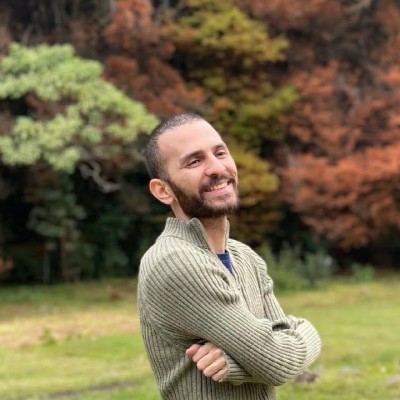
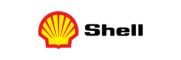