How do I use ‘type erasure’ in Swift?
Type erasure is a powerful technique in Swift used to hide the specific types of objects and provide a more abstract interface. It’s particularly useful when you want to work with different types that conform to a common protocol but need to encapsulate those types behind a unified, type-agnostic interface. Here’s how you can use type erasure in Swift:
- Create a Protocol: Begin by defining a protocol that represents the common functionality you want to expose. This protocol will serve as the public interface for your type-erased objects. For example:
```swift protocol Drawable { func draw() } ```
- Create a Type-Erased Wrapper: Next, you’ll create a type-erased wrapper (a struct or class) that conforms to the protocol and holds an instance of the concrete type that implements the protocol. This wrapper hides the concrete type behind the protocol. Here’s a simplified example using a class:
```swift class AnyDrawable: Drawable { private let drawClosure: () -> Void init<T: Drawable>(_ drawable: T) { drawClosure = drawable.draw } func draw() { drawClosure() } } ```
In this example, `AnyDrawable` can wrap any type that conforms to the `Drawable` protocol.
- Usage: You can now use `AnyDrawable` to work with different types conforming to `Drawable` without knowing their specific types:
```swift struct Circle: Drawable { func draw() { print("Drawing a circle") } } struct Square: Drawable { func draw() { print("Drawing a square") } } let circle = Circle() let square = Square() let drawableObjects: [Drawable] = [AnyDrawable(circle), AnyDrawable(square)] for drawable in drawableObjects { drawable.draw() } ```
In this example, `drawableObjects` is an array of `Drawable` instances, and it can hold objects of different types that conform to the `Drawable` protocol.
Type erasure is valuable when you want to create generic code that can work with heterogeneous types while maintaining a clear and unified interface. It’s commonly used in Swift for scenarios like collections of heterogeneous objects, UI components, and plugins where you need to abstract away the specific types involved, making your code more flexible and maintainable.
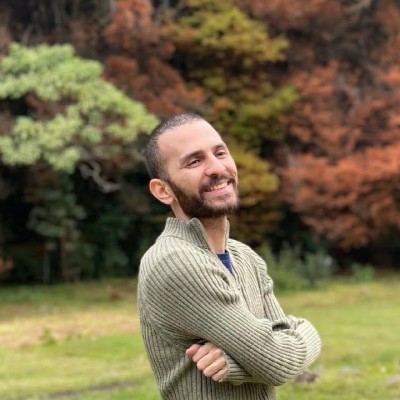
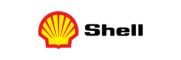