Swift Q & A
What is the difference between value types and reference types in Swift?
In Swift, one of the fundamental distinctions you’ll encounter is between value types and reference types, which determine how data is stored, copied, and shared in your code.
Value Types:
- Data Copy: Value types include basic data types like integers, floating-point numbers, and structs. When you assign a value type to a new variable or pass it as a parameter, a copy of the data is created. This means that changes made to one copy of a value type do not affect other copies.
- Immutability: Value types are often immutable, meaning their values cannot be modified after creation. This immutability ensures safety and predictability in your code.
- Copy on Assignment: When you assign a value type to another variable or pass it as an argument to a function, Swift creates a new copy of the data. This behavior is known as “copy on assignment.”
```swift var a = 5 var b = a // 'b' is a separate copy of 'a' b = 10 // 'a' remains 5 ```
Reference Types:
- Shared Data: Reference types include classes and closures. When you assign a reference type to a new variable or pass it as a parameter, you are working with a reference to the same underlying data. This means that changes made to one reference affect all other references pointing to the same data.
- Mutability: Reference types can be mutable, allowing you to change their properties or data after creation. However, this shared mutability can lead to complex interactions and potential issues like data races.
- Shared Reference: When you assign a reference type to another variable or pass it as an argument, you are creating a new reference to the same data. Both references point to the same underlying object.
```swift class Person { var name: String init(name: String) { self.name = name } }
var person1 = Person(name: "Alice") var person2 = person1 // 'person2' references the same object as 'person1' person2.name = "Bob" // 'person1' and 'person2' both have the name "Bob" ```
In summary, the key difference between value types and reference types in Swift lies in how they handle data storage and sharing. Value types create copies of data, ensuring data isolation and immutability, while reference types work with shared data, allowing for changes to be visible across all references. Understanding this distinction is crucial for effective memory management and avoiding unexpected behavior in your Swift code.
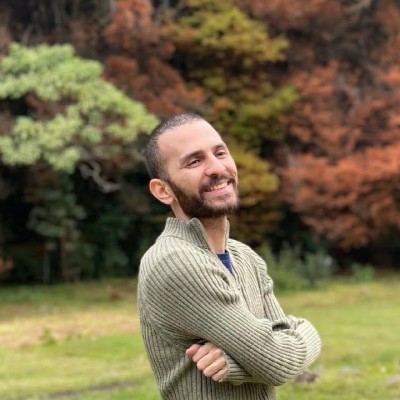
Previously at
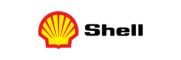
Experienced iOS Engineer with 7+ years mastering Swift. Created fintech solutions, enhanced biopharma apps, and transformed retail experiences.