How do I use the ‘where’ clause in Swift?
In Swift, the `where` clause is a powerful tool used in various contexts to filter and refine data based on specific conditions. It’s often used with collection types like arrays, dictionaries, and loops to constrain elements or iterations based on specified criteria. Here, we’ll explore how to use the `where` clause effectively in Swift.
- Filtering Arrays and Sequences:
– One common use of the `where` clause is to filter arrays and sequences. You can use it with functions like `filter(_:)` to create a new sequence that contains only the elements meeting certain conditions.
```swift let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] let evenNumbers = numbers.filter { $0 % 2 == 0 } ```
In this example, the `where` clause (in this case, represented by the condition `$0 % 2 == 0`) filters out all odd numbers, leaving only the even ones in the `evenNumbers` array.
- For-In Loops with ‘where’:
– You can use the `where` clause in `for-in` loops to iterate over elements that satisfy specific conditions.
```swift let names = ["Alice", "Bob", "Charlie", "David", "Eve"] for name in names where name.count > 4 { print(name) } ```
This loop prints only the names with a length greater than 4 characters.
- Switch Statements:
– The `where` clause can also be used in `switch` statements to add additional conditions to case clauses.
```swift let age = 30 switch age { case 0..<18: print("You are a minor.") case 18..<65 where age.isMultiple(of: 5): print("You are an adult and your age is a multiple of 5.") default: print("You are an adult.") } ```
In this example, the `where` clause narrows down the conditions for the second case, ensuring that the age is both in the range 18..<65 and a multiple of 5.
The `where` clause is a versatile feature in Swift that allows you to apply additional conditions and constraints in various contexts. Whether you’re filtering data, iterating over elements, or handling switch cases, the `where` clause provides a way to express specific criteria and make your code more precise and readable.
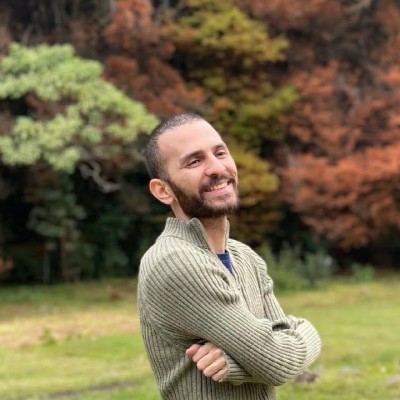
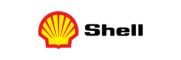