Symfony Debugging and Profiling: Tools and Techniques
As a PHP developer, you’ve probably encountered the challenges of debugging and profiling applications. When working on complex Symfony projects, these tasks can become even more daunting. Thankfully, the Symfony framework provides an array of powerful debugging and profiling tools to ease your development journey. In this blog, we’ll explore some essential tools and techniques to debug and profile Symfony applications efficiently.
1. Understanding Symfony Debugging
Before we dive into the tools, let’s understand the fundamentals of Symfony debugging. Debugging is the process of identifying and resolving errors and issues in your application’s code. Symfony offers various methods to help you find and eliminate bugs effectively.
1.1. Dump Function
The dump() function is one of the most basic yet powerful debugging tools in Symfony. It allows you to inspect variables, objects, and their properties at runtime. This function is part of the VarDumper component and provides colored and well-formatted output.
php // Example usage of dump() $variable = 'Hello, Symfony!'; dump($variable);
When executing this code, the dumped variable will be displayed with valuable information such as type, value, and file location.
1.2. Debugging with VarDumper Component
Symfony’s VarDumper component offers more advanced debugging capabilities beyond the dump() function. You can use VarDumper to customize the dumping behavior, control the maximum depth of the dumped data, and enable/disable HTML escaping for web-based debugging.
php use Symfony\Component\VarDumper\VarDumper; // Customize VarDumper settings VarDumper::setDisplayOptions([ 'maxDepth' => 5, 'maxStringLength' => 200, ]); $complexObject = new ComplexObject(); VarDumper::dump($complexObject);
1.3. Debugging Configuration
In your Symfony application, the config/packages/dev/web_profiler.yaml file contains debugging configuration. Ensure the framework section includes the profiler and web_profiler settings:
yaml framework: profiler: { only_exceptions: false } web_profiler: toolbar: true
With the toolbar enabled, you can access the profiler at the bottom of your web pages. It provides crucial information like query counts, execution time, and more.
2. Mastering Symfony Profiling
Profiling is the process of analyzing your application’s performance to identify bottlenecks and optimize its speed and resource usage. Symfony comes equipped with excellent profiling tools that can significantly enhance your application’s performance.
2.1. Web Debug Toolbar
The Symfony Web Debug Toolbar is an essential tool for profiling your application. It provides detailed information about the current request, including the time taken to render templates, executed queries, and memory usage.
To enable the toolbar, make sure your application is running in the dev environment, and the web_profiler is enabled in the config/packages/dev/web_profiler.yaml file, as mentioned earlier.
2.2. Blackfire Profiler
Blackfire Profiler is a powerful performance profiling tool that integrates seamlessly with Symfony. It helps you discover performance bottlenecks and optimize your application efficiently.
First, sign up for a Blackfire account and install the Blackfire probe on your server. Then, install the Blackfire Symfony Bundle:
bash composer require blackfire/symfony
Once installed, use the blackfire command to profile specific Symfony commands or HTTP requests:
bash # Profiling a Symfony command blackfire run bin/console some:command # Profiling an HTTP request blackfire curl http://your-app.dev/some-route
Blackfire will generate detailed reports and performance metrics, enabling you to make data-driven optimizations.
2.3. Profiling with Xdebug
Xdebug is a popular PHP extension that provides powerful profiling and debugging capabilities. Symfony seamlessly integrates with Xdebug, allowing you to profile your application and find performance bottlenecks.
To use Xdebug with Symfony, make sure it is installed and enabled on your server. Then, configure your PHP settings in php.ini:
ini zend_extension=xdebug.so xdebug.mode=profile
Now, you can use Xdebug’s profiling feature:
bash # Profiling a PHP script php -d xdebug.profiler_enable=On -d xdebug.output_dir=/tmp your-script.php
After running the script, check the generated profiling files for valuable insights into your application’s performance.
3. Techniques for Optimized Development
Optimizing your development process is crucial to maintaining a smooth workflow and delivering high-quality applications efficiently. Here are some techniques to streamline your Symfony development:
3.1. Logging and Monitoring
Symfony’s robust logging system allows you to track and record important events in your application. Proper logging helps identify issues and track the flow of execution during development and production. Combine logging with monitoring tools like New Relic or Sentry to gain deeper insights into your application’s performance and detect potential problems proactively.
yaml # config/packages/dev/monolog.yaml monolog: handlers: main: type: stream path: "%kernel.logs_dir%/%kernel.environment%.log" level: debug critical: type: slack level: critical token: '%env(SLACK_TOKEN)%' channel: '#critical-logs'
In this example, logs with a level of “critical” will be sent to a Slack channel, while all other logs will be written to a file.
3.2. Caching for Improved Performance
Symfony provides various caching mechanisms to reduce database queries and optimize performance. Use caching for frequently accessed data, such as configuration files or database results. The built-in caching components, like HttpCache, can help reduce the server load and speed up your application significantly.
php // Caching HTTP responses using HttpCache use Symfony\Bundle\FrameworkBundle\HttpCache\HttpCache; class AppCache extends HttpCache { protected function getOptions() { return [ 'default_ttl' => 3600, // Cache responses for an hour ]; } }
3.3. Optimizing Doctrine Queries
The Doctrine ORM is widely used in Symfony applications for database interactions. However, poorly optimized database queries can cause performance issues. Utilize Doctrine’s query optimization techniques, such as query caching, DQL (Doctrine Query Language), and indexed database fields.
php // Using query caching with Doctrine $entityManager->getConfiguration()->setQueryCacheImpl( new \Doctrine\Common\Cache\ArrayCache() );
Conclusion
Debugging and profiling are essential aspects of Symfony development. With the array of powerful tools and techniques explored in this blog, you can effectively identify and resolve bugs and optimize your application’s performance. Utilize Symfony’s built-in debugging features like the dump() function and VarDumper component, and leverage profiling tools like the Web Debug Toolbar, Blackfire Profiler, and Xdebug to gain deep insights into your application’s performance. Implement the outlined development optimization techniques to streamline your workflow and deliver top-notch Symfony applications with ease.
Happy debugging and profiling!
Table of Contents
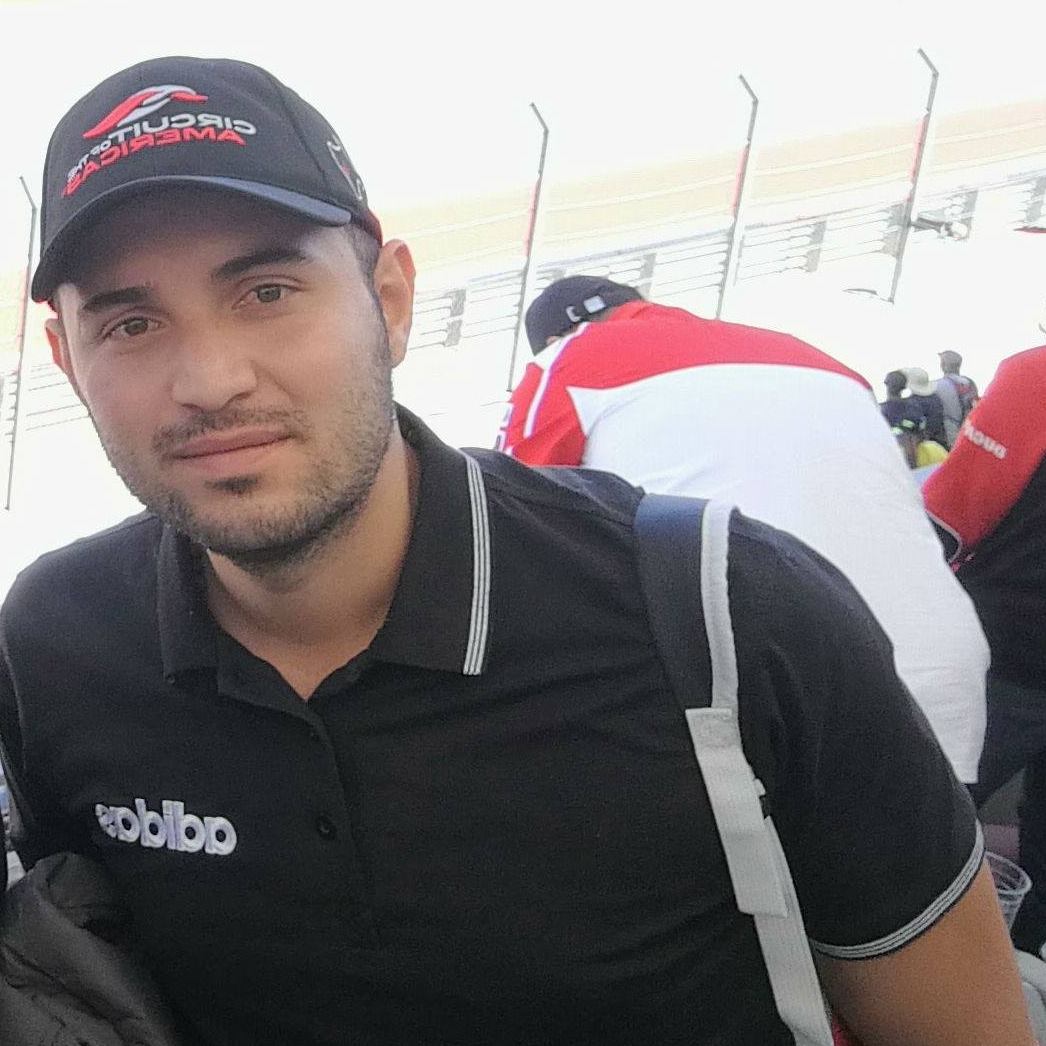
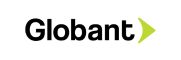