Vue.js Error Tracking with Sentry: Capturing and Monitoring Application Errors
Error tracking and monitoring are critical for maintaining the stability and performance of web applications. Sentry offers powerful tools for capturing and managing errors in real-time. This article explores how to integrate Sentry with Vue.js to enhance error tracking and monitoring capabilities.
Understanding Error Tracking in Vue.js
Error tracking involves identifying and managing errors that occur in your application. For Vue.js applications, integrating a robust error tracking system like Sentry can help you catch and fix issues before they impact your users significantly.
Setting Up Sentry for Vue.js
Sentry provides a comprehensive platform for error monitoring and performance tracking. Integrating Sentry with your Vue.js application involves several steps to ensure that errors are captured and reported correctly.
1. Installing Sentry
First, you need to add Sentry to your Vue.js project. You can install the Sentry SDK via npm:
```bash npm install @sentry/vue @sentry/tracing ```
2. Configuring Sentry in Vue.js
To configure Sentry, you’ll need to initialize it with your DSN (Data Source Name), which you can obtain from your Sentry account. Here’s how you can set it up in your Vue.js application:
```javascript // main.js import Vue from 'vue'; import App from './App.vue'; import * as Sentry from '@sentry/vue'; import { Integrations } from '@sentry/tracing'; Sentry.init({ Vue, dsn: 'https://your-public-dsn@sentry.io/your-project-id', integrations: [ new Integrations.BrowserTracing({ tracingOrigins: ['localhost', 'your-domain.com'], }), ], tracesSampleRate: 1.0, // Adjust this value based on your needs }); new Vue({ render: h => h(App), }).$mount('app'); ```
3. Capturing Errors
Once Sentry is configured, it will automatically capture unhandled errors and performance issues. However, you can also manually capture errors and custom messages as needed.
Example: Manually Capturing an Error
```javascript // SomeComponent.vue export default { methods: { throwError() { try { // Code that may throw an error throw new Error('Custom error message'); } catch (error) { Sentry.captureException(error); } }, }, }; ```
Monitoring and Analyzing Errors
Sentry provides a rich interface for monitoring and analyzing errors. You can view detailed error reports, track error frequency, and identify patterns. Use the Sentry dashboard to:
– View Error Details: See the stack trace, environment, and other context information.
– Track Error Trends: Monitor how errors change over time and identify recurring issues.
– Manage Alerts: Set up notifications for specific error thresholds or types.
Best Practices for Error Tracking with Sentry
- Fine-Tune Error Reporting: Adjust the `tracesSampleRate` and filtering settings to capture relevant errors without overwhelming your dashboard.
- Use Contextual Information: Include additional context (e.g., user information, application state) to help diagnose issues more effectively.
- Regularly Review Error Reports: Periodically check Sentry reports to address and resolve any emerging issues.
Conclusion
Integrating Sentry with Vue.js enables comprehensive error tracking and monitoring, helping you maintain application stability and improve user experience. By following the setup and best practices outlined in this guide, you can effectively capture and manage errors in your Vue.js applications.
Further Reading:
Table of Contents
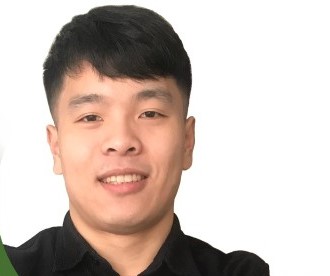
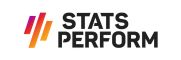