Vue.js Internationalization: Building Multilingual Web Applications
In today’s interconnected world, the demand for multilingual web applications is higher than ever. Businesses and developers are keen to reach global audiences, break language barriers, and offer a personalized experience to users from various regions. Vue.js, a progressive JavaScript framework, makes internationalization (i18n) a breeze, empowering developers to create web applications that cater to users worldwide.
Table of Contents
This blog post will guide you through the process of implementing internationalization in your Vue.js web applications. We’ll cover everything from setting up the required libraries to handling translations and language switching. So, let’s dive in!
1. What is Internationalization (i18n) in Vue.js?
Internationalization, often abbreviated as i18n, is the process of designing and adapting web applications to support multiple languages and regions without requiring major code changes. By internationalizing a Vue.js app, you make it capable of presenting content in different languages, date and time formats, currencies, and more, depending on the user’s locale or preferences.
2. Why is Internationalization Important?
In a globalized world, reaching a diverse audience is crucial for the success of any web application. Here are some key reasons why internationalization matters:
- Expanded Market Reach: By offering your app in multiple languages, you can engage with users from various regions, thereby expanding your market reach and potential customer base.
- Improved User Experience: Multilingual applications provide a more personalized experience to users, making them feel more comfortable and connected to your product.
- Higher User Retention: Users are more likely to stay and engage with your app if it speaks their native language, leading to improved user retention rates.
- Competitive Advantage: In many industries, offering a multilingual application can give you a competitive edge over competitors who cater to a single language audience.
3. Setting Up a Vue.js Project for Internationalization
Before diving into the code, let’s set up a new Vue.js project and install the necessary libraries for internationalization.
Step 1: Create a New Vue.js Project
First, make sure you have Node.js and Vue CLI installed. If not, you can install them by following the official documentation.
Now, create a new Vue.js project using Vue CLI:
bash vue create my-i18n-app cd my-i18n-app
Step 2: Install vue-i18n
Vue.js offers an excellent internationalization library called vue-i18n. Let’s install it in our project:
bash npm install vue-i18n
Step 3: Configure vue-i18n
Now that vue-i18n is installed, we need to configure it to work with our Vue.js project. Create a new directory named i18n in the src folder, and inside it, create a file named index.js:
bash mkdir src/i18n touch src/i18n/index.js
Add the following code to src/i18n/index.js:
javascript // src/i18n/index.js import Vue from 'vue'; import VueI18n from 'vue-i18n'; Vue.use(VueI18n); const messages = { // Define your translations here }; const i18n = new VueI18n({ locale: 'en', // Set the default locale here messages, }); export default i18n;
Replace messages with an object that contains translations for different languages. For example:
javascript const messages = { en: { welcome: 'Welcome to our app!', about: 'About Us', // More English translations... }, fr: { welcome: 'Bienvenue sur notre application !', about: 'À Propos', // More French translations... }, // Add translations for other languages as needed... };
Step 4: Integrate i18n with Vue.js App
Next, let’s integrate the vue-i18n instance we created into our main Vue.js app. Open the main.js file in the src folder and add the following code:
javascript // src/main.js import Vue from 'vue'; import App from './App.vue'; import i18n from './i18n'; new Vue({ i18n, // Inject i18n into Vue instance render: (h) => h(App), }).$mount('#app');
With this setup, our Vue.js app is now ready for internationalization. In the next section, we’ll see how to use translations in our components.
4. Translating Content in Vue.js Components
In Vue.js, translating content is straightforward using the $t method provided by vue-i18n. Let’s see how to use it in our components.
Step 1: Display Translations in Templates
In your Vue component templates, you can use the $t method to display translated content:
html <template> <div> <h1>{{ $t('welcome') }}</h1> <p>{{ $t('about') }}</p> </div> </template>
In this example, the text inside {{ $t(…) }} will be dynamically replaced with the corresponding translation based on the current locale.
Step 2: Language Switching
To enable language switching in your app, you need to add a language switcher component and handle the language change events. Here’s a basic implementation:
html <template> <div> <select v-model="currentLanguage" @change="changeLanguage"> <option v-for="lang in supportedLanguages" :key="lang" :value="lang">{{ lang }}</option> </select> </div> </template> <script> export default { data() { return { currentLanguage: this.$i18n.locale, supportedLanguages: ['en', 'fr'], // Add more languages as needed }; }, methods: { changeLanguage() { this.$i18n.locale = this.currentLanguage; }, }, }; </script>
In this example, we create a select dropdown that allows the user to choose their preferred language. The currentLanguage data property is bound to the select element, and when the user selects a different language, the changeLanguage method is called to update the locale in vue-i18n.
Conclusion
Congratulations! You’ve learned the basics of implementing internationalization in Vue.js web applications. By following the steps outlined in this guide, you can create powerful multilingual apps that cater to users worldwide, breaking language barriers and offering a more personalized experience.
Remember to continually improve your app’s internationalization by expanding the translations, handling date and number formatting, and addressing potential localization issues. Happy coding and best of luck with your multilingual Vue.js journey!
Table of Contents
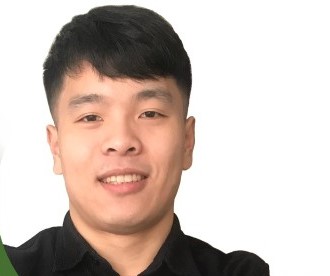
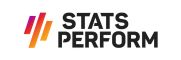