Mastering the Art of Hiring Vue.js Developers: A Comprehensive Interview Questions Guide
Vue.js, the progressive JavaScript framework, has revolutionized front-end development. To create exceptional web applications, hiring skilled Vue.js developers is essential. This guide serves as your compass through the hiring process, empowering you to assess candidates’ technical acumen, problem-solving abilities, and Vue.js expertise. Let’s embark on this journey, uncovering essential interview questions and strategies to identify the perfect Vue.js developer for your team.
Table of Contents
1. How to Hire Vue.js Developers
Preparing to hire Vue.js developers? Follow these steps for a successful hiring journey:
- Job Requirements: Define specific job requirements, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ Vue.js proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of Vue.js Developers to Look For
When assessing Vue.js developers, look out for these key skills:
- Vue.js Mastery: A strong understanding of Vue.js syntax, components, directives, and state management.
- Front-End Proficiency: Familiarity with HTML, CSS, and JavaScript, and their integration with Vue.js.
- Component Reusability: Experience in building reusable Vue components for creating dynamic user interfaces.
- State Management: Proficiency in using Vuex for managing application state in complex Vue.js applications.
- Routing and Navigation: Skill in implementing routing and navigation for seamless user experiences.
- Vue CLI Expertise: Familiarity with Vue CLI for project scaffolding and rapid development.
- Problem-Solving Skills: Ability to tackle intricate problems and devise efficient solutions.
3. Overview of the Vue.js Developer Hiring Process
Here’s a high-level overview of the Vue.js developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create captivating job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting Vue.js Developer Interview Questions
Develop a comprehensive set of interview questions covering Vue.js intricacies, problem-solving aptitude, and relevant technologies.
4. Sample Vue.js Developer Interview Questions and Answers
Let’s explore sample questions with detailed answers to assess candidates’ Vue.js skills:
Q1. Explain the concept of Vue.js directives and provide examples of built-in directives.
A: Directives in Vue.js are special tokens in the markup that instruct the library to do something to a DOM element. Examples of built-in directives are:
- v-model: Enables two-way data binding between form input and component data.
<input v-model="message"> <p>{{ message }}</p>
- v-for: Renders a list of items based on an array.
<ul> <li v-for="item in items">{{ item }}</li> </ul>
- v-bind: Dynamically binds an attribute to an expression.
<a v-bind:href="url">Link</a>
Q2. What is Vue Router, and how is it used for routing in Vue.js applications?
A: Vue Router is the official routing library for Vue.js. It allows you to implement single-page application navigation by defining routes and components associated with each route.
import Vue from 'vue'; import VueRouter from 'vue-router'; Vue.use(VueRouter); const routes = [ { path: '/', component: Home }, { path: '/about', component: About }, // ... other routes ]; const router = new VueRouter({ routes, });
Q3. Write a Vue.js component that fetches data from an API and displays it in a template.
<template> <div> <ul> <li v-for="item in items">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { items: [], }; }, mounted() { this.fetchData(); }, methods: { async fetchData() { const response = await fetch('https://api.example.com/data'); this.items = await response.json(); }, }, }; </script>
Q4. Explain the concept of Vue.js computed properties and provide an example.
A: Computed properties in Vue.js are properties that are automatically recalculated when their dependent properties change. They are useful for performing calculations or transformations.
<template> <div> <p>Length of message: {{ messageLength }}</p> </div> </template> <script> export default { data() { return { message: 'Hello, Vue!', }; }, computed: { messageLength() { return this.message.length; }, }, }; </script>
By incorporating these technical questions and answers into your interview process, you’ll be well-equipped to evaluate candidates’ Vue.js proficiency and their ability to work with the framework. These questions cover a range of essential Vue.js concepts, ensuring that you assess candidates’ capabilities comprehensively.
Q5. Explain the concept of Vue.js reactive data binding and provide an example.
A: Reactive data binding in Vue.js allows you to automatically update the view whenever the underlying data changes. An example:
<template> <div> <p>Message: {{ message }}</p> <input v-model="message"> </div> </template> <script> export default { data() { return { message: 'Hello, Vue!', }; }, }; </script>
Q6. How do you manage application-wide state in Vue.js applications?
A: Vuex is the recommended state management solution for Vue.js applications. It allows you to centralize and manage the state of your application in a predictable and organized manner.
Q7. Explain the purpose of Vue.js lifecycle hooks and provide examples of commonly used hooks.
A: Lifecycle hooks are special methods that allow you to perform actions at different stages of a component’s lifecycle. Examples of commonly used hooks are created
, mounted
, updated
, and destroyed
.
Q8. Write a Vue.js directive that highlights an element when clicked.
<template> <div v-highlight>Click me to highlight</div> </template> <script> Vue.directive('highlight', { bind(el) { el.style.cursor = 'pointer'; el.addEventListener('click', () => { el.style.backgroundColor = 'yellow'; }); }, }); </script>
Q9. Explain the concept of Vue.js slots and provide an example of using named slots.
A: Vue.js slots allow you to compose components with flexible content. Named slots allow you to specify where particular content should be placed.
Q10. What are mixins in Vue.js, and why are they used?
A: Mixins in Vue.js are a way to reuse component options. They allow you to encapsulate and share component logic, making it easier to maintain and extend functionality across multiple components.
Q11. How does Vue.js handle animations and transitions?
A: Vue.js provides built-in support for adding animations and transitions to elements when they enter, leave, or move within the DOM. This is achieved through the transition
component and CSS classes.
Q12. Explain the concept of Vue.js watchers and provide an example.
A: Watchers in Vue.js allow you to react to changes in a specific data property. An example:
<template> <div> <p>Count: {{ count }}</p> <button @click="increment">Increment</button> </div> </template> <script> export default { data() { return { count: 0, }; }, methods: { increment() { this.count++; }, }, watch: { count(newValue, oldValue) { console.log(`Count changed from ${oldValue} to ${newValue}`); }, }, }; </script>
Q13. Write a Vue.js component that emits a custom event when a button is clicked.
<template> <div> <button @click="emitEvent">Click me</button> </div> </template> <script> export default { methods: { emitEvent() { this.$emit('custom-event', 'Event data'); }, }, }; </script>
Q14. Explain the concept of Vue.js scoped CSS and provide an example.
A: Scoped CSS in Vue.js allows you to encapsulate styles within a component, preventing them from affecting other components. An example:
<template> <div class="container"> <p class="text">This is a scoped paragraph.</p> </div> </template> <style scoped> .container { background-color: lightgray; } .text { font-weight: bold; } </style>
Q15. How do you optimize the performance of Vue.js applications?
A: Performance optimization in Vue.js involves techniques such as lazy loading, using asynchronous components, minimizing re-renders with shouldComponentUpdate
, and utilizing the built-in keep-alive
component.
5. Hiring Vue.js Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected Vue.js developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By harnessing the expertise of CloudDevs, you can effortlessly identify and hire exceptional Vue.js developers, ensuring your team possesses the skills required to build extraordinary web applications.
6. Conclusion
Equipped with these additional technical questions and insights, you’re now well-prepared to assess Vue.js developers’ prowess comprehensively. Whether you’re crafting dynamic user interfaces or pioneering front-end innovations, securing the right Vue.js developers for your team is the cornerstone of your project’s triumph.
Table of Contents
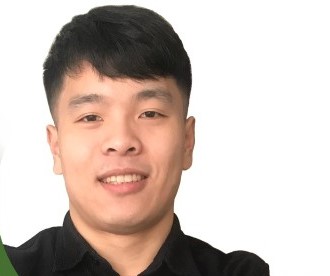
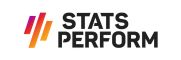