Vue.js and Mapbox Integration: Building Interactive Maps
In today’s digital age, interactive maps have become an integral part of web applications across various industries, from e-commerce to logistics and beyond. Incorporating maps into your Vue.js applications can greatly enhance user experience and provide valuable functionality. One powerful tool for creating interactive maps is Mapbox, a mapping platform that allows developers to build custom maps tailored to their specific needs. In this article, we’ll explore how to integrate Mapbox into Vue.js applications to create stunning and interactive maps.
Getting Started with Vue.js and Mapbox Integration
Before we dive into the integration process, let’s ensure we have everything set up to get started:
- Vue.js Project Setup: If you haven’t already, set up a Vue.js project using Vue CLI or your preferred method.
- Mapbox Account: Sign up for a Mapbox account to access their APIs and services. You’ll need an access token, which you can obtain from the Mapbox dashboard.
- Vue-mapbox Package: Install the vue-mapbox package, which provides Vue.js bindings for Mapbox GL JS, the JavaScript library used for rendering interactive maps.
Once you have these prerequisites in place, we can proceed with integrating Mapbox into our Vue.js application.
Integrating Mapbox into Vue.js
1. Install vue-mapbox Package
npm install @mapbox/mapbox-gl-vue
2. Set Up Mapbox Access Token
In your Vue.js project, create a .env file and add your Mapbox access token:
VUE_APP_MAPBOX_ACCESS_TOKEN=your-access-token-here
3. Initialize Mapbox Component
Create a new Vue component for your map, and import the necessary modules:
<template> <div ref="map" class="map-container"></div> </template> <script> import mapboxgl from 'mapbox-gl'; import Mapbox from '@mapbox/mapbox-gl-vue'; export default { name: 'MapComponent', components: { Mapbox, }, data() { return { map: null, }; }, mounted() { mapboxgl.accessToken = process.env.VUE_APP_MAPBOX_ACCESS_TOKEN; this.map = new mapboxgl.Map({ container: this.$refs.map, style: 'mapbox://styles/mapbox/streets-v11', center: [-74.5, 40], zoom: 9, }); }, }; </script> <style scoped> .map-container { height: 400px; width: 100%; } </style>
4. Customize Mapbox Styles and Features
You can customize your map’s style, add markers, layers, and interactive features using Mapbox GL JS APIs. Refer to the Mapbox documentation for detailed guidance on map customization.
Adding Interactivity and Functionality
Now that you have your basic map set up, you can enhance it with interactivity and functionality tailored to your application’s requirements. Here are a few examples of what you can do:
Adding Markers
Place markers on the map to indicate specific locations or points of interest. You can customize marker icons, popups, and interactions.
Drawing Tools
Allow users to draw shapes, lines, or polygons on the map. This feature is useful for applications involving route planning, geofencing, or spatial analysis.
Geocoding and Search
Implement geocoding functionality to convert addresses or place names into geographic coordinates, enabling users to search for locations on the map.
Conclusion
In this tutorial, we’ve covered the basics of integrating Mapbox into Vue.js applications to create interactive maps. By following these steps and exploring the various features and customization options offered by Mapbox, you can build dynamic and engaging mapping experiences for your users.
To learn more about Mapbox integration and advanced features, check out the Mapbox documentation and Vue-mapbox GitHub repository.
Happy mapping!
External Resources
Remember to replace your-access-token-here with your actual Mapbox access token.
Table of Contents
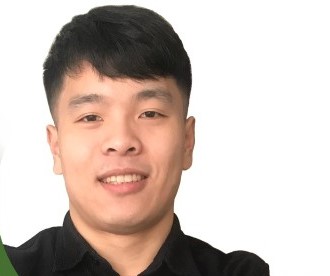
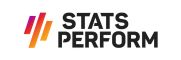