Vue.js Progressive Image Loading: Enhancing Performance and User Experience
In the ever-evolving landscape of web development, enhancing performance and user experience (UX) is paramount. One crucial aspect of this is image loading, as images often constitute a significant portion of a webpage’s content. Slow image loading can frustrate users and adversely affect engagement. Vue.js, with its robust ecosystem and flexibility, offers a solution to this challenge through progressive image loading techniques.
Understanding Progressive Image Loading
Progressive image loading is a strategy that involves initially displaying a low-resolution placeholder image and gradually replacing it with higher-resolution versions as they load. This approach prioritizes the visual experience by providing users with immediate feedback while the full-quality image loads in the background. Vue.js provides several methods to implement progressive image loading efficiently.
Implementation with Vue-Lazyload
Vue-Lazyload is a popular Vue.js plugin that simplifies the implementation of lazy loading, including progressive image loading. By incorporating Vue-Lazyload into your Vue.js project, you can effortlessly optimize image loading performance. Let’s delve into a basic example:
<template> <img v-lazy="imageSrc" src="placeholder.jpg" alt="Placeholder Image"> </template> <script> import VueLazyload from 'vue-lazyload'; export default { data() { return { imageSrc: 'high-resolution-image.jpg', }; }, directives: { lazy: VueLazyload, }, }; </script>
In this example, the v-lazy directive binds the imageSrc to the image element, while the src attribute points to a placeholder image. As the high-resolution image loads, it seamlessly replaces the placeholder, providing a smooth user experience.
Leveraging Intersection Observer
Another approach to progressive image loading involves leveraging the Intersection Observer API, which allows you to asynchronously observe changes in the intersection of an element with its containing parent or viewport. Vue.js makes it easy to integrate Intersection Observer into your applications:
<template> <img :src="imageSrc" alt="Progressive Image" ref="imageRef"> </template> <script> export default { data() { return { imageSrc: 'placeholder.jpg', }; }, mounted() { const options = { root: null, rootMargin: '0px', threshold: 0.5, }; const observer = new IntersectionObserver(this.handleIntersect, options); observer.observe(this.$refs.imageRef); }, methods: { handleIntersect(entries) { entries.forEach(entry => { if (entry.isIntersecting) { this.imageSrc = 'high-resolution-image.jpg'; } }); }, }, }; </script>
In this example, the IntersectionObserver is used to detect when the image enters the viewport (isIntersecting). Once it does, the imageSrc is updated with the high-resolution image, progressively enhancing the user experience.
Real-World Examples
To further illustrate the effectiveness of Vue.js progressive image loading, let’s explore some real-world implementations:
Unsplash
Unsplash, the popular stock photography platform, utilizes progressive image loading to deliver stunning visuals without sacrificing performance, ensuring a seamless browsing experience for users.
Medium
Medium, a renowned publishing platform, employs progressive image loading to enhance the readability of articles, keeping readers engaged by minimizing loading times.
Pinterest leverages progressive image loading to showcase a vast array of visual content, allowing users to discover and save inspiration effortlessly.
Conclusion
Vue.js progressive image loading is a powerful technique for optimizing performance and improving user experience in web applications. By implementing strategies such as Vue-Lazyload and Intersection Observer, developers can ensure that images load efficiently, keeping users engaged and satisfied. Embracing these techniques empowers web developers to create compelling and high-performing Vue.js applications that stand out in today’s competitive digital landscape.
Table of Contents
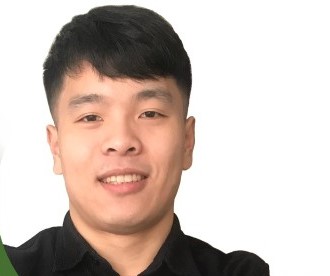
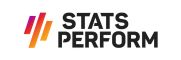